Tuples
A tuple is a collection which is ordered and unchangeable or immutable.
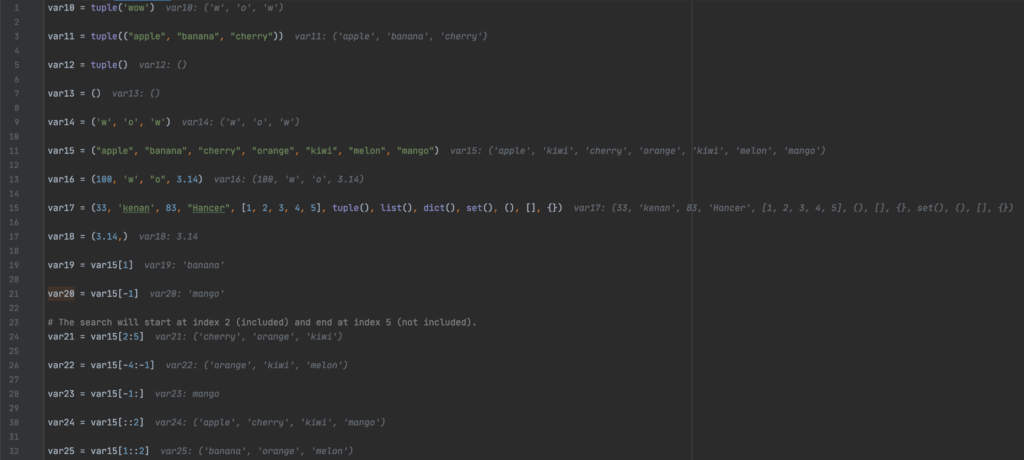
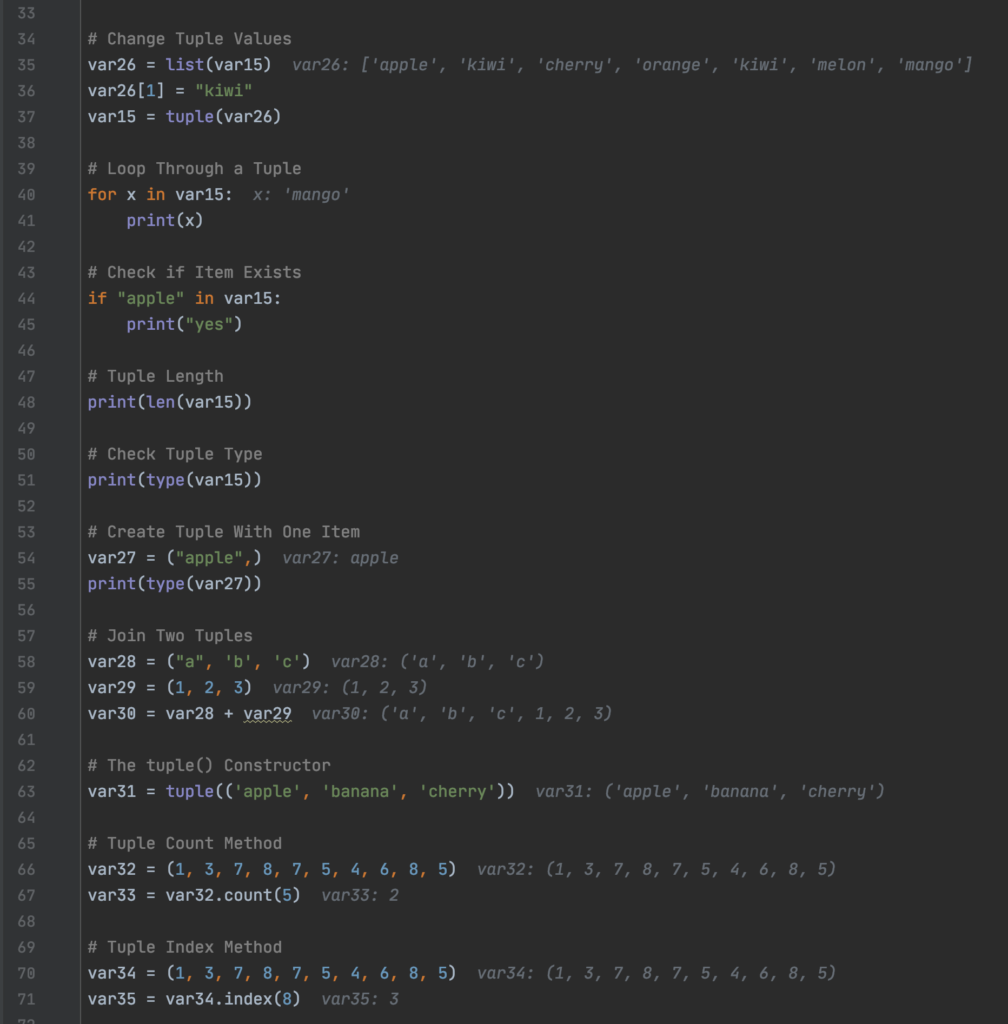
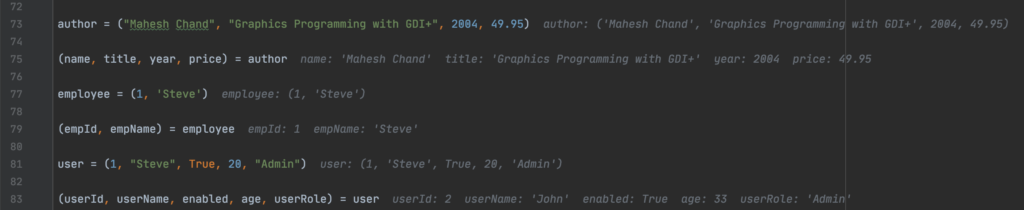
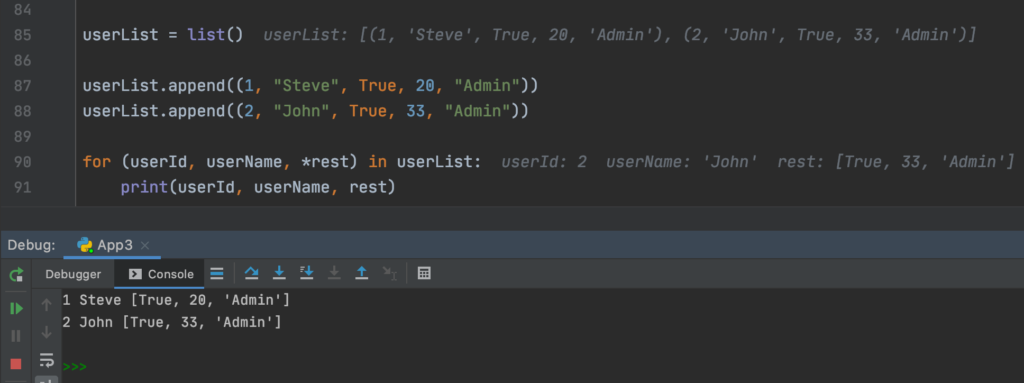
In order to find more details about this
usage, follow link below.
https://www.w3schools.com/Js/js_this.asp
I just took the following part from that page.
I try to understand difference between function expression
, shorthand method definition
, arrow function
and bind()
.
Notice that I defined sayGoodBye()
function in constructor, it means that whenever i create a new instance from this Person
class, sayGoodBye()
function will be defined for every instance.
sayHello()
function is defined as a class property. But, as you can see in the following screenshot, class properties are also defined in constructor so class properties are just a sugar syntax.
getFullName()
function is defined as shorthand method definition. But, Babel didn't move function definition in class constructor. So, if we use shorthand method definition in class, it will be a prototype function .
It mean they are only defined once, instead of once per instance.
Notice that in below screenshot, getFullName()
function is in prototype of Person class, but sayHello()
and sayGoodBye()
are not.
There is one more post about Promise and Async/Await usage in the following link.
There are 3 ways to handle asynchronous function calls.
Mocha is a test framework to run tests.
https://mochajs.org/#assertions
Chai is an assertion library and you can find full documentation in the following link.
https://www.chaijs.com/api/bdd/
There are three type of assertion libraries in Chai.
expect
and should
use the same chainable language to construct assertions.
Assume that expect library is used like below
expect(firstName).to.be.a('string');
expect(firstName).to.equal('kenan');
expect(firstName).to.have.lengthOf(5);
expect(beverages).to.have.property('tea').with.lengthOf(3);
Above code could be written with should library like below.
firstName.should.be.a('string');
firstName.should.equal('kenan');
firstName.should.have.lengthOf(5);
beverages.should.have.property('tea').with.lengthOf(3);
You can find more detailed usage with Express, Mocha and Chai in the following repository
https://github.com/nodejs-projects-kenanhancer/express-demo.git
Continue readingFindIntersection(strArr) read the array of strings stored in strArrwhich will contain 2 elements: the first element will represent a list of comma-separated numbers sorted in ascending order, the second element will represent a second list of comma-separated numbers (also sorted). Your goal is to return a comma-separated string containing the numbers that occur in elements of strArr in sorted order. If there is no intersection, return the string false.
You can see examples in the pictures and more demo codes in the rest of post.
I just want to create some demo for enum to create a builder . I improved this concept step by step in demos. Notice that all the codes are doing same job but in a different way.