I try to understand difference between function expression
, shorthand method definition
, arrow function
and bind()
.
Notice that I defined sayGoodBye()
function in constructor, it means that whenever i create a new instance from this Person
class, sayGoodBye()
function will be defined for every instance.
sayHello()
function is defined as a class property. But, as you can see in the following screenshot, class properties are also defined in constructor so class properties are just a sugar syntax.
getFullName()
function is defined as shorthand method definition. But, Babel didn't move function definition in class constructor. So, if we use shorthand method definition in class, it will be a prototype function .
It mean they are only defined once, instead of once per instance.
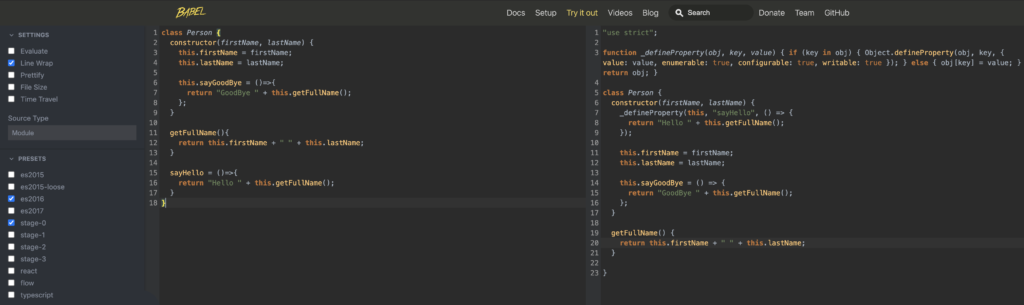
Notice that in below screenshot, getFullName()
function is in prototype of Person class, but sayHello()
and sayGoodBye()
are not.
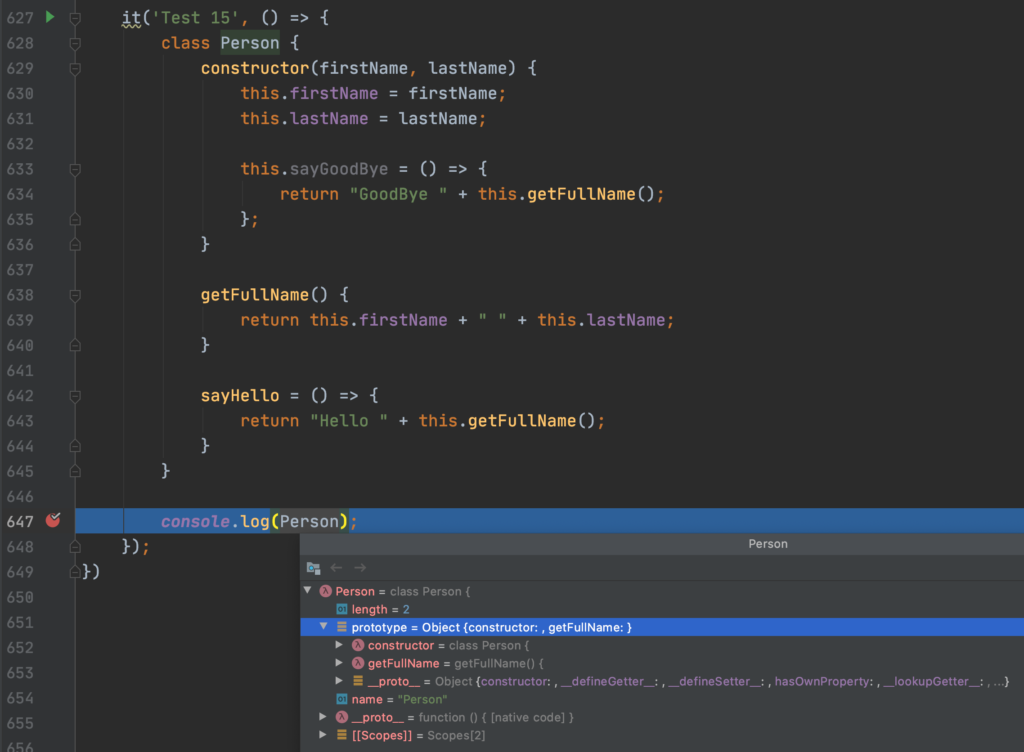
Demo 1 (ES6 shorthand method)
getFullName()
is ES6 shorthand method definition usage.
Demo 2 (function expression)
Demo 1 code could be written like below.
Demo 3
Demo 4
Demo 5 (bind()
usage)
As you can see in line 45 and 46, I used bind()
method. So that when Collection
add()
method is called, it will call onAdd()
method in Dictionary
and lastly onAdd()
method can call log()
method. But if we didn't use bind()
method then onAdd()
wouldn't call log()
method because this
would be undefined
.
Ok i think you can see in the following screenshot detaily. I removed bind()
method in line 223. After that run the code in debug mode. this
value is undefined
in line 229.
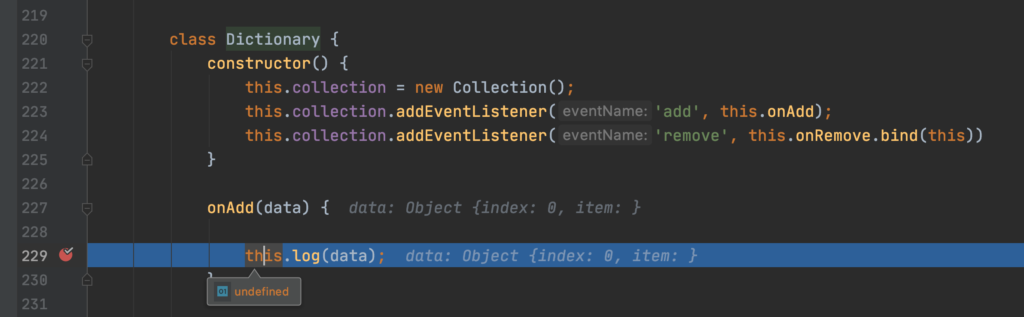
Ok i put bind()
method in line 223, so this
value is Dictionary
in line 229.
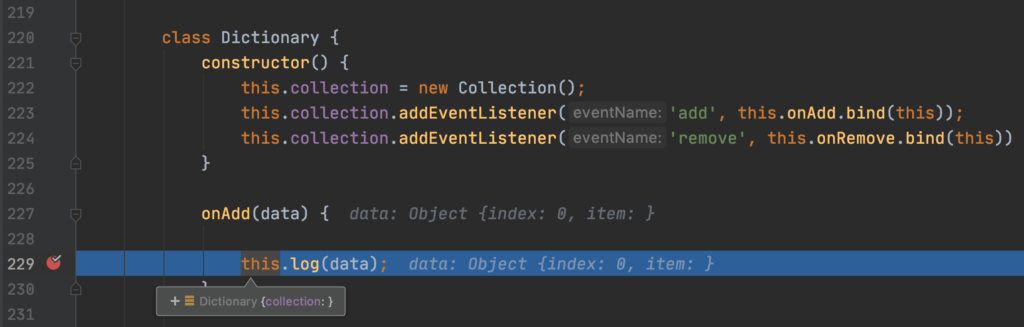
The following code shows usage of bind()
method.
Demo 6 (usage of arrow function instead of bind()
)
I wrap onAdd()
and onRemove()
methods with arrow function. Because arrow functions capture enclosing context (or outher function or closure).
As you can see in the following screenshot, this
value is Dictionary
in debug mode.
This way is best way so that we don't need to use bind()
function. (bind()
function is slower way because it creates a new wrapper function)
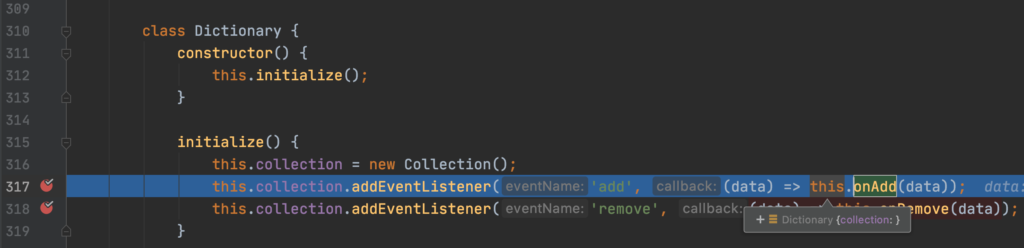
This is the full code with arrow function
Demo 7 (arrow function as class property)
I used arrow function in class directly as shown in line 415 and 420 for onAdd()
and onRemove()
methods
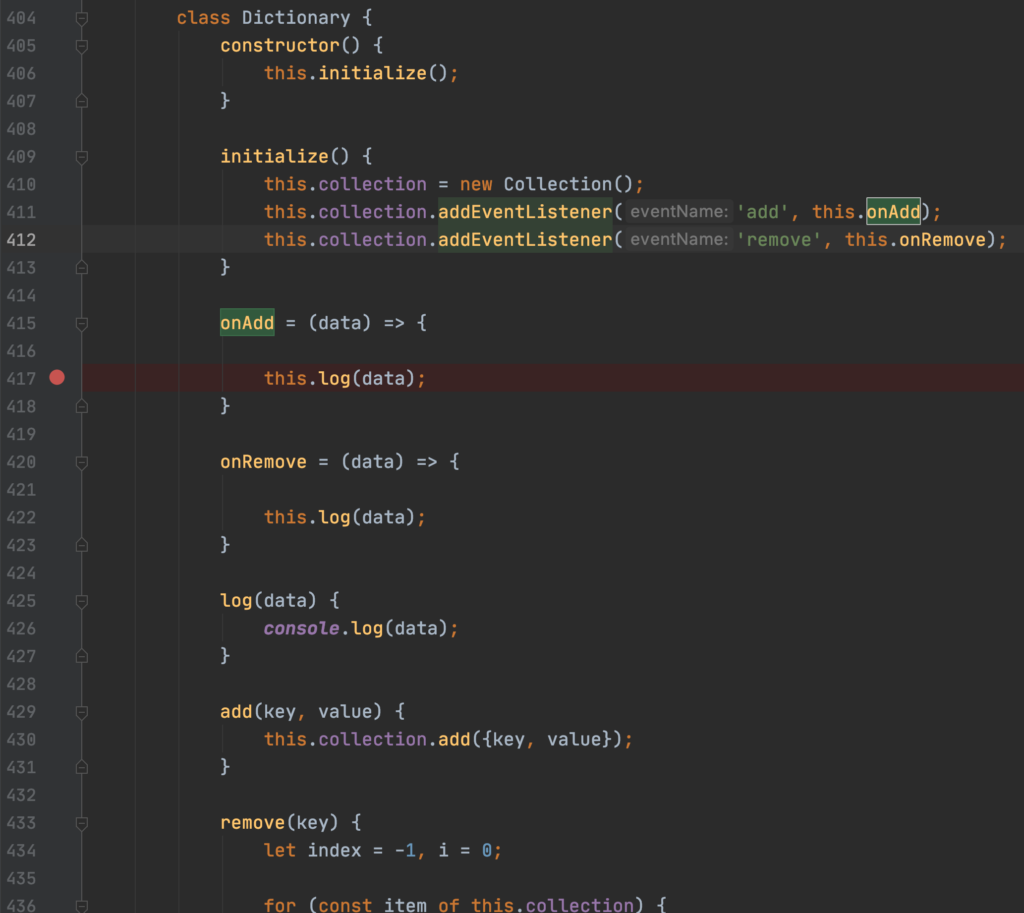
The following code is same as above code. just onAdd()
and onRemove()
functions are created as arrow function in the constructor.
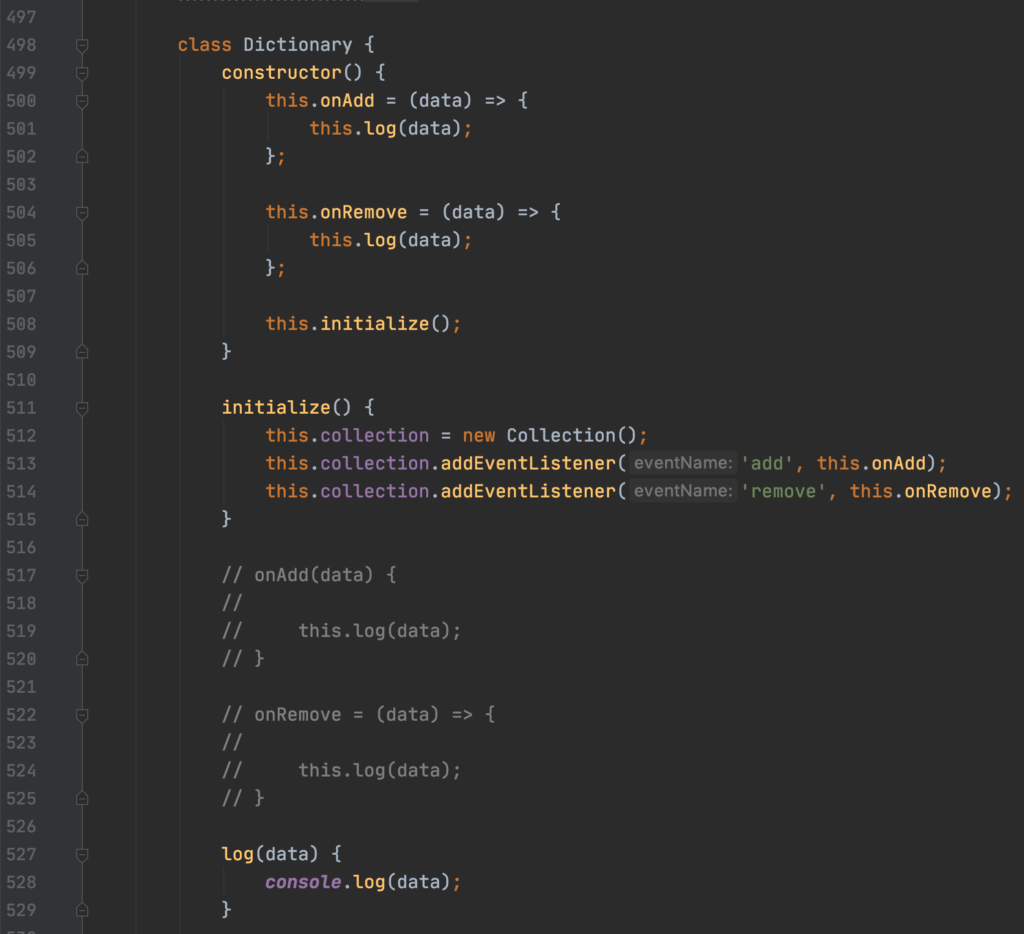
Using arrow functions instead of shorthand functions cause loosing onAdd()
and onRemove()
from prototype
. Arrow functions are anonymous functions. So both two usages like above screenshots don't have arrow functions in prototype
of Dictionary class.
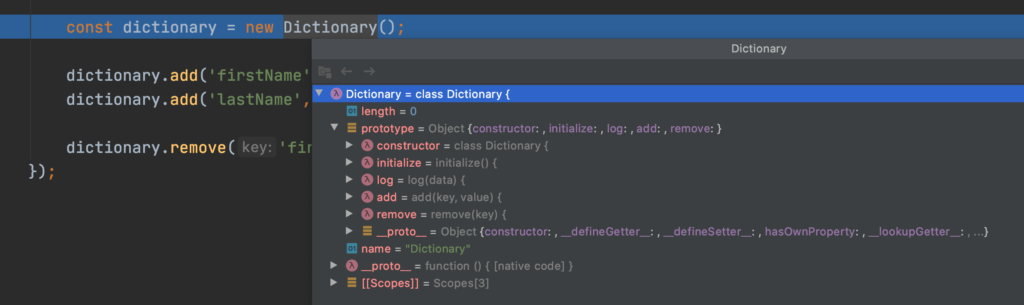