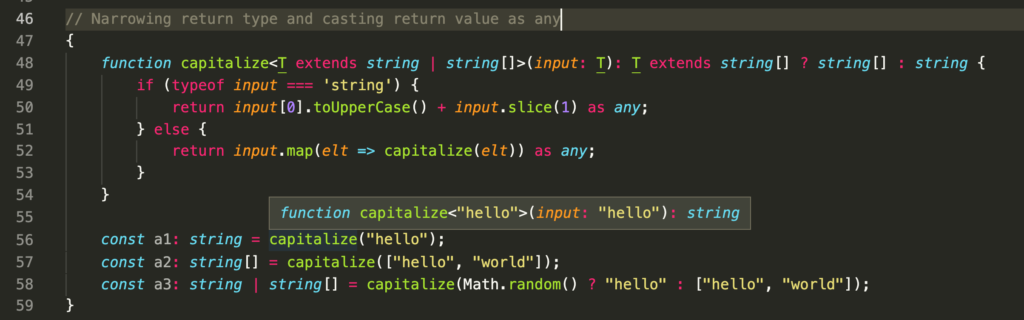
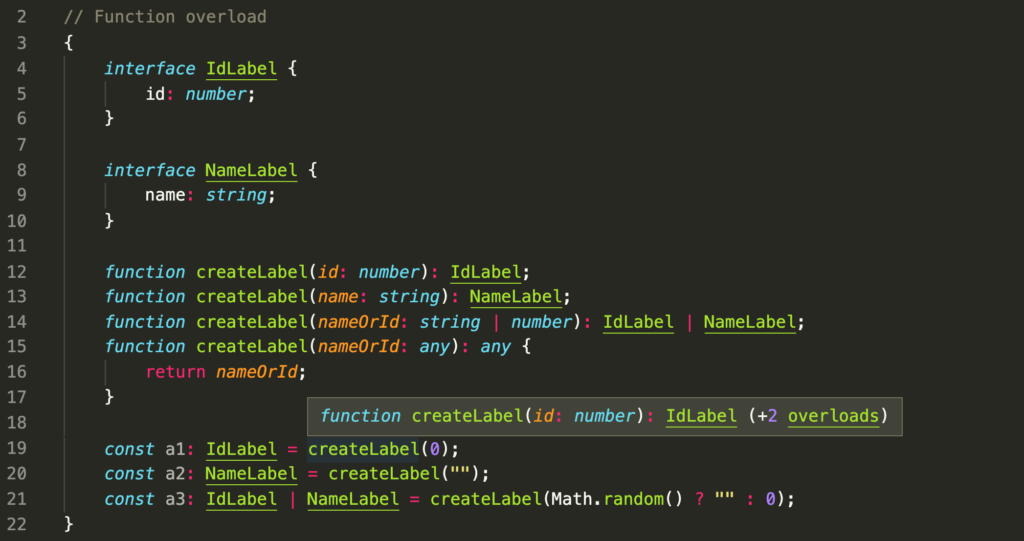
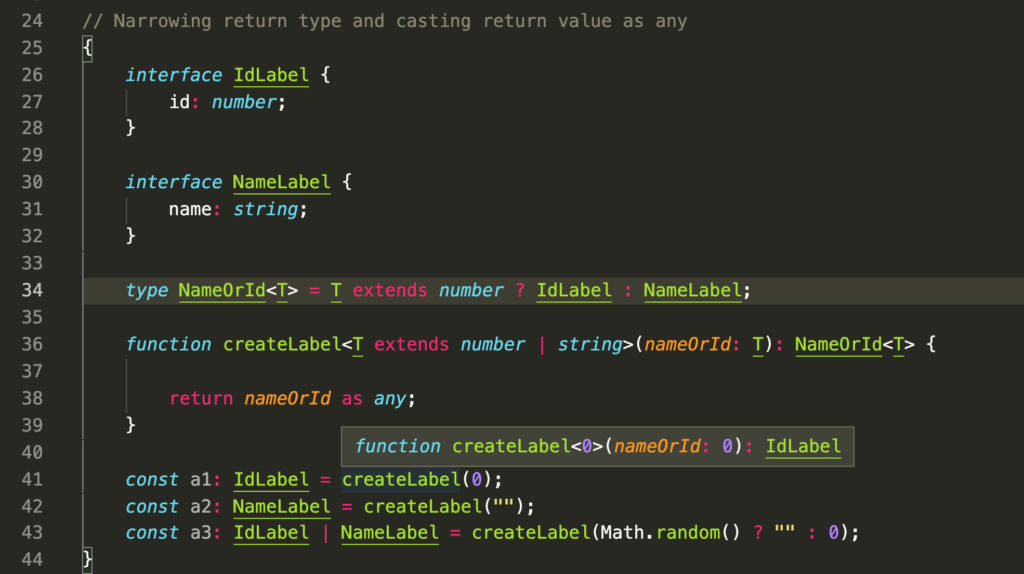
Narrowing return type of function is a little bit strange in TypeScript. So I share use cases below from bad to good 🙂
Notice that even I call foo(0)
in line 8, when I hover mouse on foo
in line 8, popup shows val parameter type as string | number
union type. But it should show as number. So it is not narrowing parameter and return type of function.
This usage narrows parameter and return type of function as literal type. But we need to narrow to type of parameter not value of parameter as literal type. Popup shows generic parameter type as 0
, that's why, parameter and return type becomes 0
.
The aim of this code is simply to show related fields in data
field dependent on eventType
.
When ChangePassword
is selected in eventType
filed, then userName
, oldPassword
and newPassword
are becoming as required fields.
When ForgotPassword
is selected in eventType
field, then userName
is just becoming required.
const msg1 = publishMessage?.({
eventType: 'ChangePassword',
data: {
userName: '',
oldPassword: '',
newPassword: '',
},
subject: '',
topic: '',
});
const msg2 = publishMessage?.({
eventType: 'ChangePassword_Success',
data: {
emailSubject: 'Password Verification code is ######',
emailMessage: '',
},
subject: '',
topic: '',
});
const msg3 = publishMessage?.({
eventType: 'ForgotPassword',
data: {
userName: ''
},
subject: '',
topic: '',
});
const msg4 = publishMessage?.({
eventType: 'ForgotPassword_Success',
data: {
resetPasswordVerificationCode: '',
emailSubject: '',
emailMessage: ''
},
subject: '',
topic: '',
});
Continue reading Notice that addTodoV2
and addTodoV3
error for extra field WRONG_FIELD
, but addTodoV1
doesn't error for extra field in line 45.