Mocha is a test framework to run tests.
https://mochajs.org/#assertions
Chai is an assertion library and you can find full documentation in the following link.
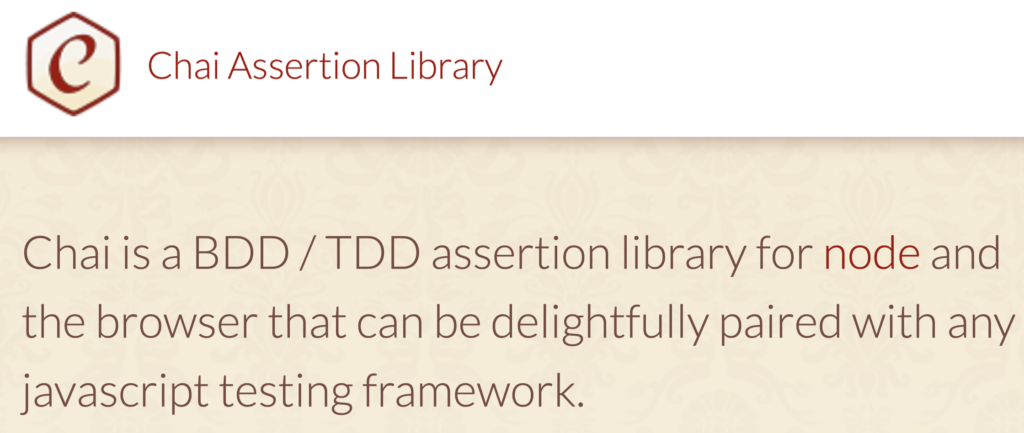
https://www.chaijs.com/api/bdd/
There are three type of assertion libraries in Chai.
- Expect
- Should
- Assert
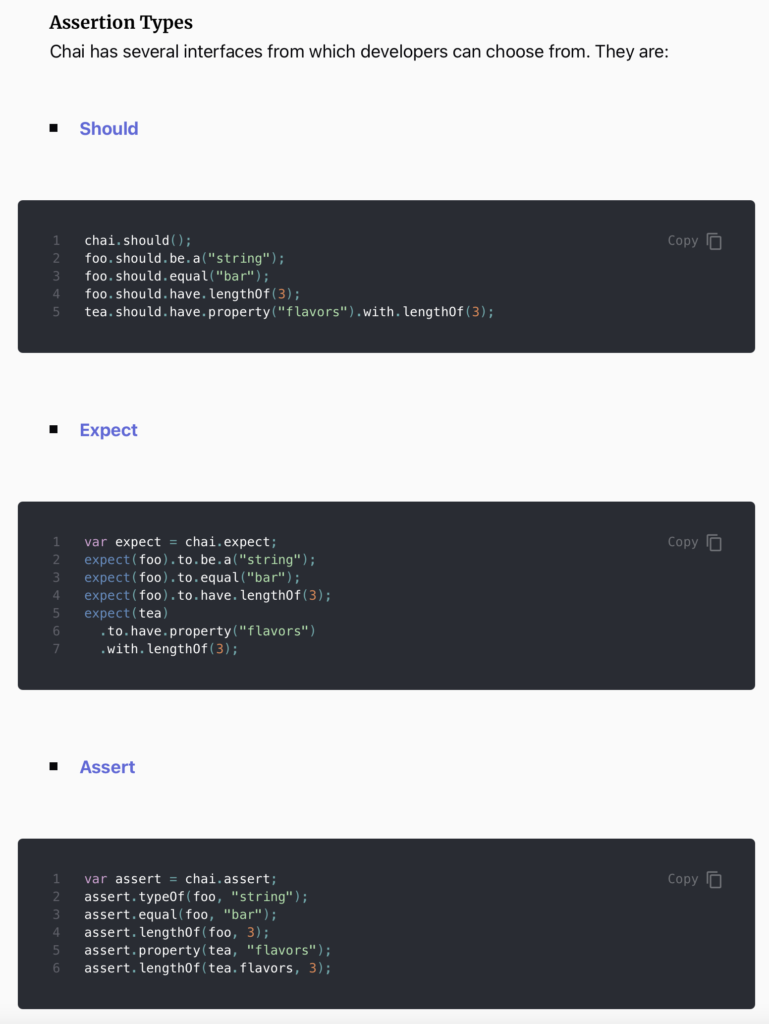
expect
and should
use the same chainable language to construct assertions.
Assume that expect library is used like below
expect(firstName).to.be.a('string');
expect(firstName).to.equal('kenan');
expect(firstName).to.have.lengthOf(5);
expect(beverages).to.have.property('tea').with.lengthOf(3);
Above code could be written with should library like below.
firstName.should.be.a('string');
firstName.should.equal('kenan');
firstName.should.have.lengthOf(5);
beverages.should.have.property('tea').with.lengthOf(3);
You can find more detailed usage with Express, Mocha and Chai in the following repository
https://github.com/nodejs-projects-kenanhancer/express-demo.git
You can find all the usages in the following code.
const chai = require('chai');
const { expect, assert } = chai;
const should = chai.should();
const firstName = 'kenan';
const beverages = { tea: [ 'chai', 'matcha', 'oolong' ] };
describe('Chai Expect Usage',()=>{
it('Expect Simple Usage',()=>{
expect(firstName).to.be.a('string');
expect(firstName).to.equal('kenan');
expect(firstName).to.have.lengthOf(5);
expect(beverages).to.have.property('tea').with.lengthOf(3);
});
});
describe('Chai Should Usage', ()=>{
it('Should Simple Usage', ()=>{
firstName.should.be.a('string');
firstName.should.equal('kenan');
firstName.should.have.lengthOf(5);
beverages.should.have.property('tea').with.lengthOf(3);
});
});
describe('Chai Assert Usage', ()=>{
it('Assert Simple Usage',()=>{
assert.typeOf(firstName, 'string');
assert.typeOf(firstName, 'string','firstName is a string');
assert.equal(firstName, 'kenan', 'firstName equal `kenan`');
assert.lengthOf(firstName, 5, 'firstName`s value has a length of 5');
assert.lengthOf(beverages.tea, 3, 'beverages has 3 types of tea');
});
});
describe('pow', function(){
it('raises to n-th power', function(){
assert.equal(Math.pow(2,3), 8);
expect(Math.pow(2,3)).to.equal(8);
Math.pow(2,3).should.equal(8);
});
});
describe('Array', ()=>{
describe('#indexOf', ()=>{
it('it should return -1 when the value is not present', ()=>{
const myArray = [1,2,3];
assert.isArray(myArray, 'is array of numbers');
assert.include(myArray, 2, 'array contains 2');
assert.lengthOf(myArray, 3, 'array contains 3 numbers');
assert.equal(myArray.indexOf(4), -1);
expect(myArray).to.be.an('array').that.includes(2);
expect(myArray).to.have.lengthOf(3);
expect(myArray.length).to.equal(3);
myArray.should.be.an('array').that.includes(2);
myArray.should.have.lengthOf(3);
myArray.length.should.equal(3);
});
});
});
Same code can be executed in the following repl editor.To see unit tests open myTest.js
file.
More examples are in the following demo. To see unit tests open myTest.js
file.