Tuples
A tuple is a collection which is ordered and unchangeable or immutable.
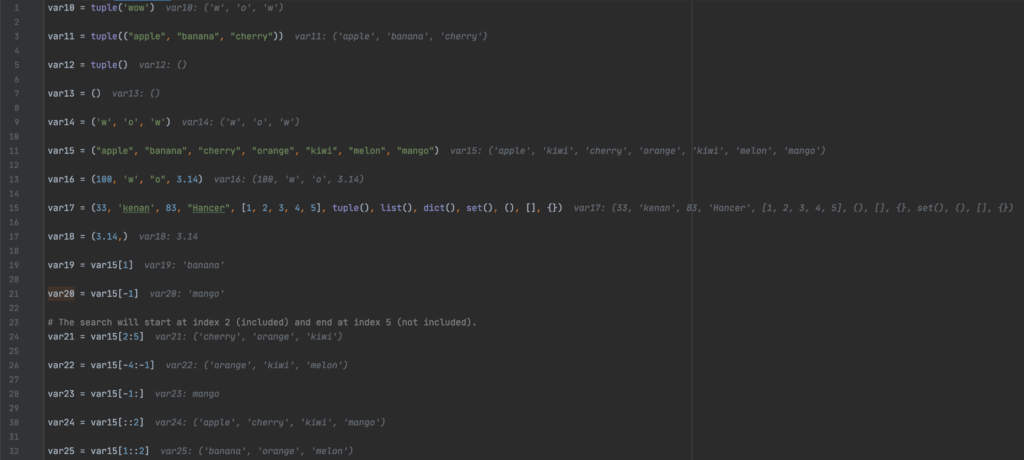
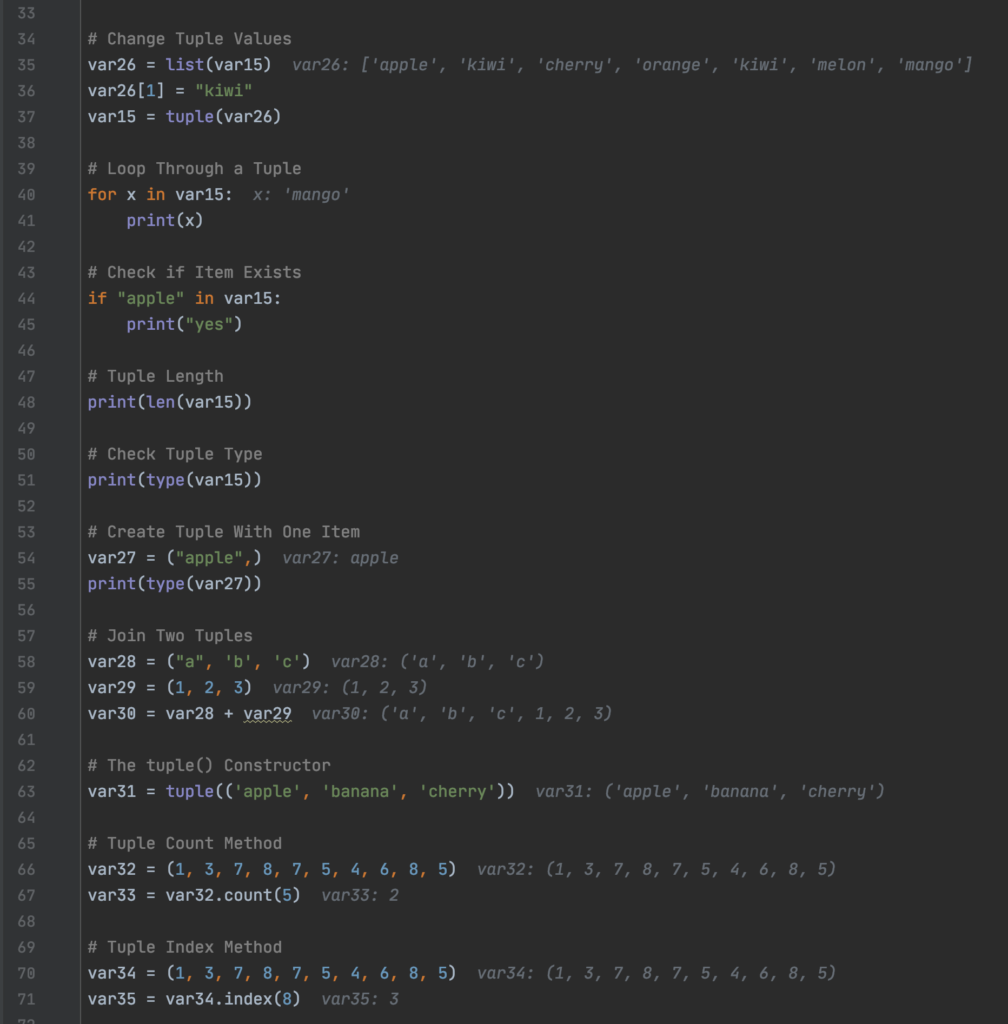
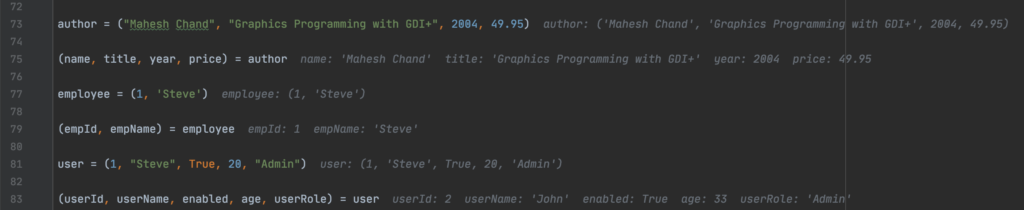
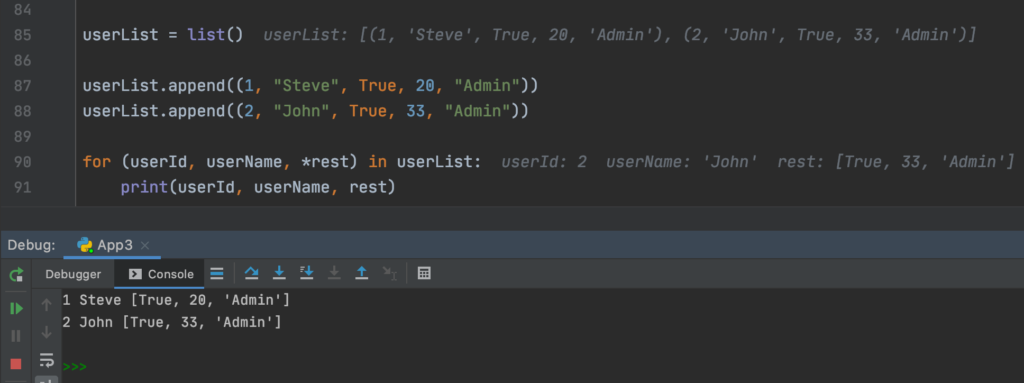
Once a tuple is created, you cannot change its values. Tuples are unchangeable, or immutable as it also is called.
But there is a workaround. You can convert the tuple into a list, change the list, and convert the list back into a tuple.