Mocha is a test framework to run tests.
https://mochajs.org/#assertions
Chai is an assertion library and you can find full documentation in the following link.
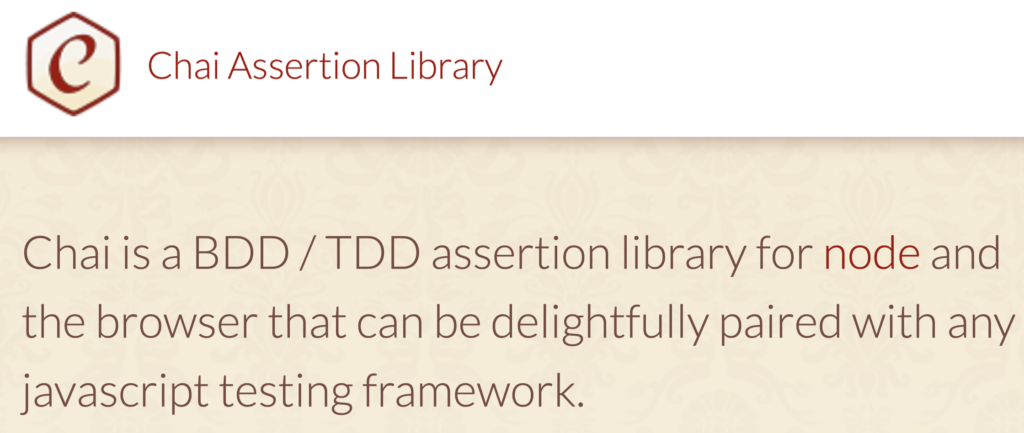
https://www.chaijs.com/api/bdd/
There are three type of assertion libraries in Chai.
- Expect
- Should
- Assert
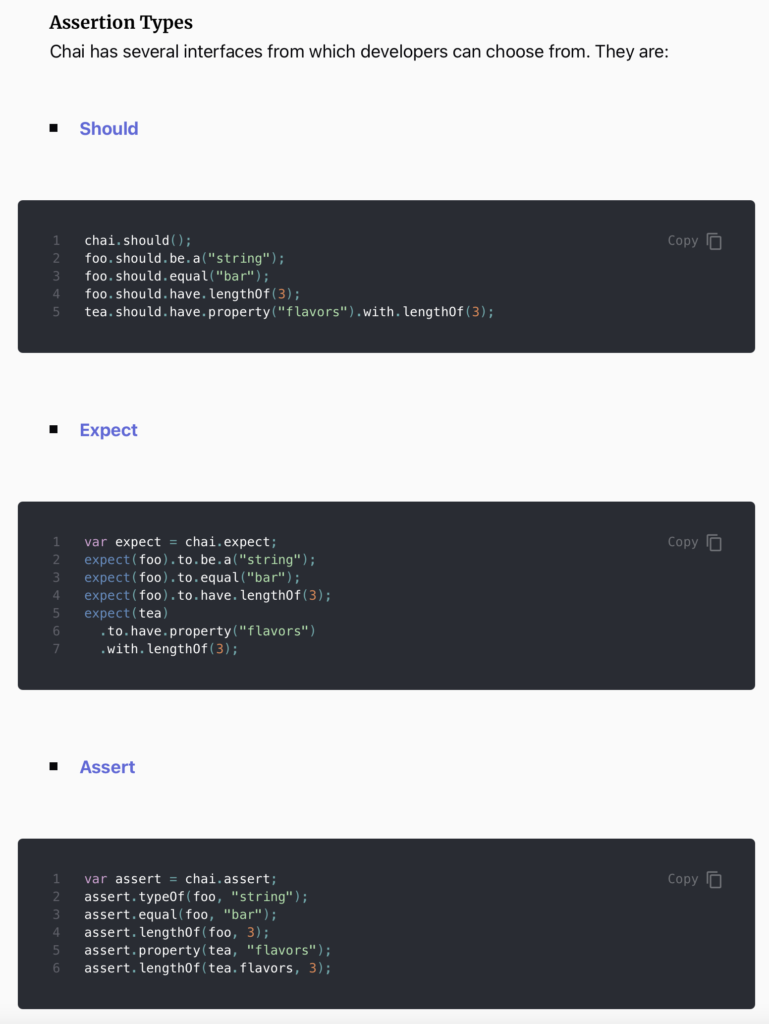
expect
and should
use the same chainable language to construct assertions.
Assume that expect library is used like below
expect(firstName).to.be.a('string');
expect(firstName).to.equal('kenan');
expect(firstName).to.have.lengthOf(5);
expect(beverages).to.have.property('tea').with.lengthOf(3);
Above code could be written with should library like below.
firstName.should.be.a('string');
firstName.should.equal('kenan');
firstName.should.have.lengthOf(5);
beverages.should.have.property('tea').with.lengthOf(3);
You can find more detailed usage with Express, Mocha and Chai in the following repository
https://github.com/nodejs-projects-kenanhancer/express-demo.git
Continue reading