I try to understand difference between function expression
, shorthand method definition
, arrow function
and bind()
.
Notice that I defined sayGoodBye()
function in constructor, it means that whenever i create a new instance from this Person
class, sayGoodBye()
function will be defined for every instance.
sayHello()
function is defined as a class property. But, as you can see in the following screenshot, class properties are also defined in constructor so class properties are just a sugar syntax.
getFullName()
function is defined as shorthand method definition. But, Babel didn't move function definition in class constructor. So, if we use shorthand method definition in class, it will be a prototype function .
It mean they are only defined once, instead of once per instance.
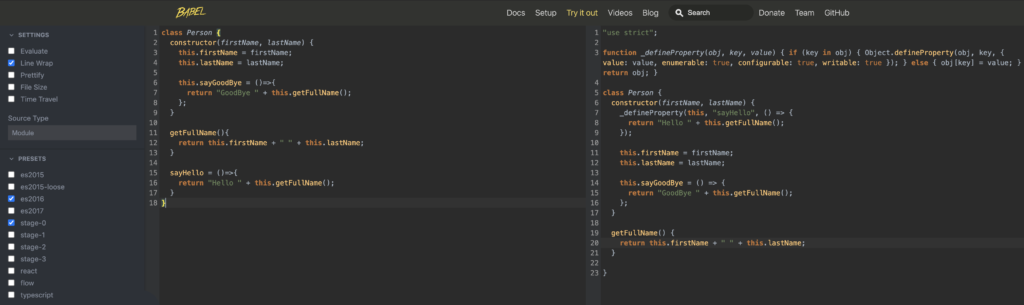
Notice that in below screenshot, getFullName()
function is in prototype of Person class, but sayHello()
and sayGoodBye()
are not.