I sometimes try to remember basis of JavaScript Functions. So, I often exercise about it. I followed this link https://www.w3schools.com/js/js_function_definition.asp. I copied some important descriptions from that link. My aim is to follow this post when I need to remember again 🙂
JavaScript Functions
A JavaScript function is a block of code designed to perform a particular task.
A Function is much the same as a Procedure or a Subroutine, in other programming languages.
Why Functions?
You can reuse code: Define the code once, and use it many times.
You can use the same code many times with different arguments, to produce different results.
JavaScript Function Types
- Function Declaration (function statement)
- Function Expression
- Function Constructor
- Self-Invoking Function (Immediately Invokable Function Expression IIFE)
- Arrow Function
- Object Method Shorthand Definition
- Generator Function
JavaScript Function Syntax
Function parameters are the names listed in the function definition.
Function arguments are the real values passed to (and received by) the function.
1. Function Declaration (function statement)
When a function declaration is created, a variable named as function name is created as well. This variable is hoisted top of the current scope. In other words, variable is moved to top of current scope. This means that the function can be called before the function declaration
2. Function Expression
The function below is actually an anonymous function (a function without a name).
Functions stored in variables do not need function names. They are always invoked (called) using the variable name.
3. Function() Constructor
Functions can also be defined with a built-in JavaScript function constructor called Function()
.
4. Self-Invoking Function (Immediately Invokable Function Expression IIFE)
When functions are used only once, a common pattern is an IIFE (Immediately Invokable Function Expression).
You have to add parentheses around the function to indicate that it is a function expression:
The function below is actually an anonymous self-invoking function (function without name).
This example is about closure usage. It shows that there is a x variable in counter IIFE and it is accessible and by inner function so that when we call counter function (actually we are calling returned function), x will be global for inner function. In other words, x will be in closure of inner function 🙂
I just want to show function closure in debug time. It is more clear for developers (it is like that for me :))
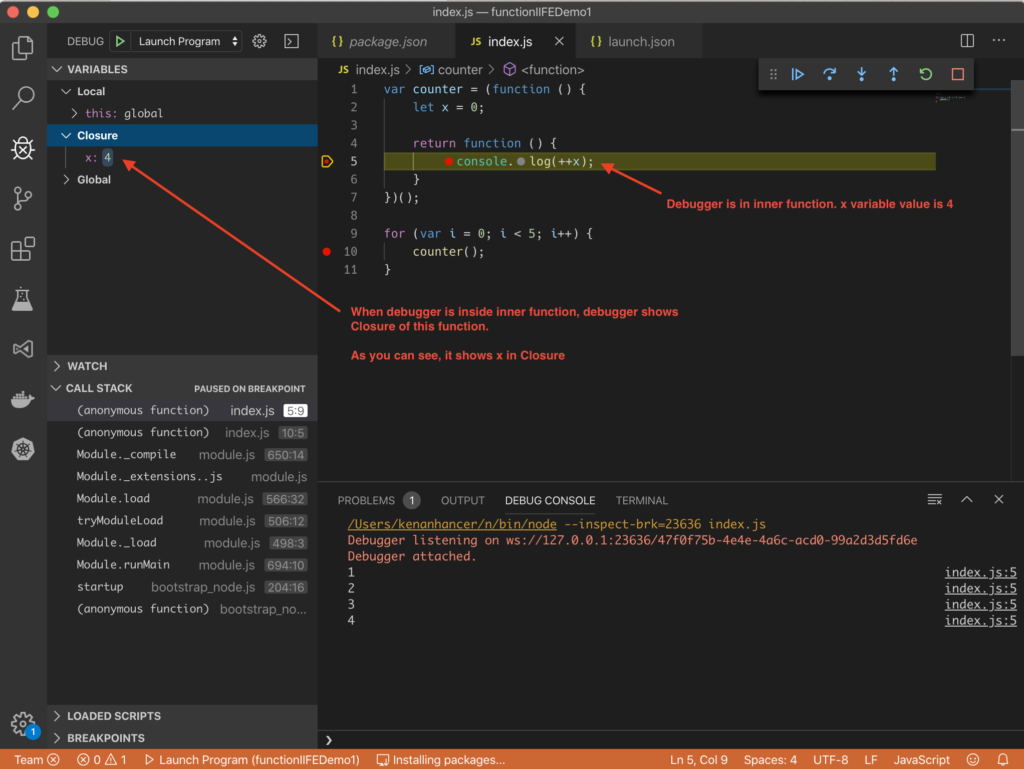
5. Arrow Function
Arrow functions allows a short syntax for writing function expressions.
It has a shorter syntax compared to function expressions and does not have its own this
, arguments, super, or new.target. Arrow functions are always anonymous.
If you need to see this in a function same as the enclosing context (or outer function), then prefer to use arrow function easily 🙂
Other example is the following code.
6. Object Method Shorthand Definition
It is used in a method declaration on object literals, ES6 classes, computed property names and methods.
7. Generator Function
A generator is a special type of function which can stop its execution midway and then start from the same point after some time. Generators are a combination of functions and iterators.
Generators are defined as a function with an asterisk(*) beside the function.
function* name(arguments) { statements }
or
function *name(arguments) { statements }
it is returning the following value.
{ value: value, done: true|false }
follow this link (https://javascript.info/generators) for more information.