This post is just a reminder for me. So in order to find more details about dotnet CLI, follow the below link
https://docs.microsoft.com/en-us/dotnet/core/tools/dotnet
$ dotnet new console -h
Console App (C#)
Author: Microsoft
Description: A project for creating a command-line application that can run on .NET on Windows, Linux and macOS
Usage:
dotnet new console [options] [template options]
Options:
-n, --name <name> The name for the output being created. If no name is specified, the name of the output directory is used.
-o, --output <output> Location to place the generated output.
--dry-run Displays a summary of what would happen if the given command line were run if it would result in a template creation.
--force Forces content to be generated even if it would change existing files.
--no-update-check Disables checking for the template package updates when instantiating a template.
--project <project> The project that should be used for context evaluation.
-lang, --language <C#> Specifies the template language to instantiate.
--type <project> Specifies the template type to instantiate.
Template options:
-f, --framework <net5.0|net6.0|net7.0> The target framework for the project.
Type: choice
net7.0 Target net7.0
net6.0 Target net6.0
net5.0 Target net5.0
Default: net7.0
--langVersion <langVersion> Sets the LangVersion property in the created project file
Type: text
--no-restore If specified, skips the automatic restore of the project on create.
Type: bool
Default: false
--use-program-main Whether to generate an explicit Program class and Main method instead of top-level statements.
Type: bool
Default: false
To see help for other template languages (F#, VB), use --language option:
dotnet new console -h --language F#
Creating a C# Project
$ dotnet new --list
These templates matched your input:
Template Name Short Name Language Tags
-------------------------------------------- ------------------ ---------- --------------------------------
ASP.NET Core Empty web [C#],F# Web/Empty
ASP.NET Core gRPC Service grpc [C#] Web/gRPC
ASP.NET Core Web API webapi [C#],F# Web/WebAPI
ASP.NET Core Web App webapp,razor [C#] Web/MVC/Razor Pages
ASP.NET Core Web App (Model-View-Controller) mvc [C#],F# Web/MVC
ASP.NET Core with Angular angular [C#] Web/MVC/SPA
ASP.NET Core with React.js react [C#] Web/MVC/SPA
ASP.NET Core with React.js and Redux reactredux [C#] Web/MVC/SPA
Blazor Server App blazorserver [C#] Web/Blazor
Blazor Server App Empty blazorserver-empty [C#] Web/Blazor/Empty
Blazor WebAssembly App blazorwasm [C#] Web/Blazor/WebAssembly/PWA
Blazor WebAssembly App Empty blazorwasm-empty [C#] Web/Blazor/WebAssembly/PWA/Empty
Class Library classlib [C#],F#,VB Common/Library
Console App console [C#],F#,VB Common/Console
dotnet gitignore file gitignore Config
Dotnet local tool manifest file tool-manifest Config
EditorConfig file editorconfig Config
global.json file globaljson Config
MSBuild Directory.Build.props file buildprops MSBuild/props
MSBuild Directory.Build.targets file buildtargets MSBuild/props
MSTest Test Project mstest [C#],F#,VB Test/MSTest
MVC ViewImports viewimports [C#] Web/ASP.NET
MVC ViewStart viewstart [C#] Web/ASP.NET
NuGet Config nugetconfig Config
NUnit 3 Test Item nunit-test [C#],F#,VB Test/NUnit
NUnit 3 Test Project nunit [C#],F#,VB Test/NUnit
Protocol Buffer File proto Web/gRPC
Razor Class Library razorclasslib [C#] Web/Razor/Library
Razor Component razorcomponent [C#] Web/ASP.NET
Razor Page page [C#] Web/ASP.NET
Solution File sln,solution Solution
Web Config webconfig Config
Worker Service worker [C#],F# Common/Worker/Web
xUnit Test Project xunit [C#],F#,VB Test/xUnit
$ dotnet --list-sdks
5.0.408 [/Users/kenanhancer/.dotnet/sdk]
6.0.411 [/Users/kenanhancer/.dotnet/sdk]
7.0.305 [/Users/kenanhancer/.dotnet/sdk]
Creating a Console Project
Use any template short name
after dotnet net
and .NET SDK version after --framework
option.
$ dotnet new console --framework net6.0 -o console-demo1
Creating a WebApi Project
$ dotnet new webapi --framework net6.0 -o webapi-todo-demo1
Specify .NET version in project folder
If you have multiple versions of the .NET Core SDK installed, the version that's used can be controlled using a global.json
file. This file allows you to specify which version of the .NET Core SDK should be used.
$ dotnet new globaljson --sdk-version 6.0.411 --force
1. Creating a C# Console Project
-o or –output is location to place for new generated project.
$ dotnet new console --framework net6.0 --output enum_demo4
# OR
$ dotnet new console --framework net6.0 -o enum_demo4
# OR
$ dotnet new console --framework net6.0 --name enum_demo4

Folder Structure
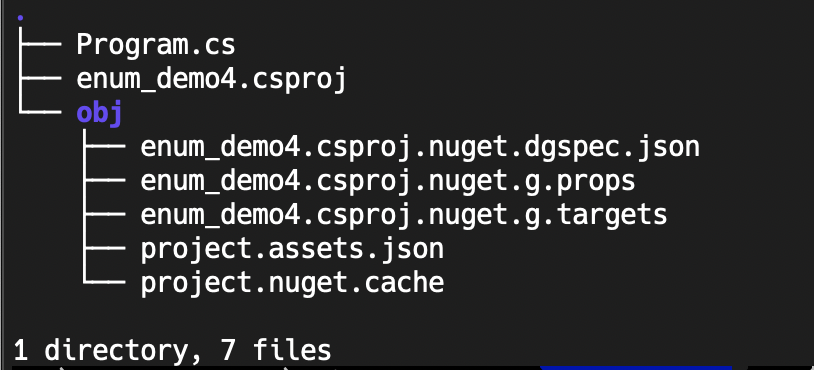
Generated Project
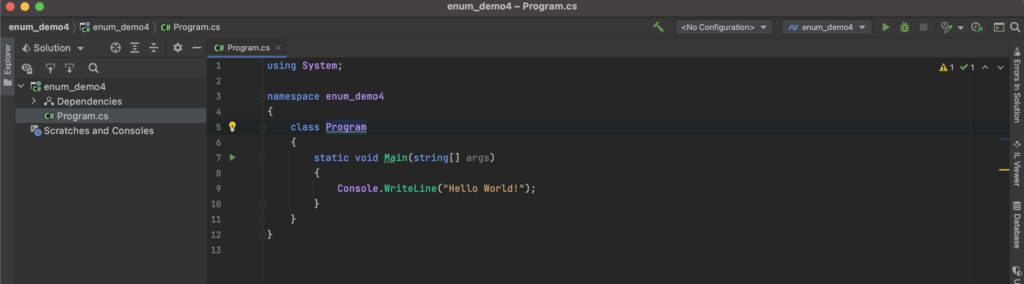
2. Building – Compiling Project
Build the project in the current directory. It build project and its dependencies.
$ dotnet build
Build the specified project
$ dotnet build ./demo_app1
Build project and its dependencies using Release configuration
$ dotnet build --configuration Release
3. Running
Run the project in the current directory:
$ dotnet run
Run the specified project
$ dotnet run --project ./demo_app1/demo_app1.csproj
or
$ dotnet run -p./demo_app1/demo_app1.csproj
4. Cleaning
Clean the project in the current directory:
$ dotnet clean
Clean specified project:
$ dotnet clean ./demo_app1