Primitive Types (Boolean, Number, String)
Boolean
let isDone: boolean = false;
Number
let decimal: number = 6;
let hex: number = 0xf00d;
let binary: number = 0b1010;
let octal: number = 0o744;
let big: bigint = 100n;
String
let color: string = "blue";
let fullName: string = 'Bob Bobbington';
let age: number = 37;
let sentence: string = `Hello, my name is ${fullName}.
I'll be ${age + 1} years old next month.`;
Arrays
let list: number[] = [1, 2, 3];
let list2: Array<number> = [1, 2, 3];
Tuples
const x: [string, number] = ['hello', 10];
x[0] = 'world';
x[1] = 20;
console.log(`Hello ${x[0]}, ${x[1]}`);
const [a, b] = x;
const a1: Array<string | number> = [...x];
const a2 = [...x];
const a3: [string, number, Date] = ['hello', 11, new Date()];
const [greeting, age, birthDate] = a3;
const a4: [Record<PropertyKey, any>, (p: Record<PropertyKey, any>) => void] = [{}, (p) => { }];
const [counter, setCounter] = a4;
setCounter({ ...counter, name: 'kenan' });
Enums
enum Color {
Red,
Green,
Blue,
}
let c: Color = Color.Green;
enum Color {
Red = 1,
Green = 2,
Blue = 4,
}
let c: Color = Color.Green;
enum Color {
Red = 1,
Green,
Blue,
}
let colorName: string = Color[2];
// Displays 'Green'
console.log(colorName);
const enum Suit {
Clubs,
Diamonds,
Hearts,
Spades
}
const a1 = Suit.Spades; // emits const a1 = 3;
enum MyFlags {
None = 0,
Neat = 1,
Cool = 2,
Awesome = 4,
Best = Neat | Cool | Awesome
}
const a2 = MyFlags.Best; // emits const a2 = 7;
Union Types
function printId(id: number | string) {
if (typeof id === "string") {
// In this branch, id is of type 'string'
console.log(id.toUpperCase());
} else {
// Here, id is of type 'number'
console.log(id);
}
}
// OK
printId(101);
// OK
printId("202");
function welcomePeople(x: string[] | string) {
if (Array.isArray(x)) {
// Here: 'x' is 'string[]'
console.log("Hello, " + x.join(" and "));
} else {
// Here: 'x' is 'string'
console.log("Welcome lone traveler " + x);
}
}
Type Aliases
type ID = number | string;
type PrimitiveArray = Array<string | number | boolean>;
type MyNumber = number;
type Callback = () => void;
type Point = {
x: number;
y: number;
};
// Exactly the same as the earlier example
function printCoord(pt: Point) {
console.log("The coordinate's x value is " + pt.x);
console.log("The coordinate's y value is " + pt.y);
}
printCoord({ x: 100, y: 100 });
Interfaces
interface Point {
x: number;
y: number;
}
function printCoord(pt: Point) {
console.log("The coordinate's x value is " + pt.x);
console.log("The coordinate's y value is " + pt.y);
}
printCoord({ x: 100, y: 100 });
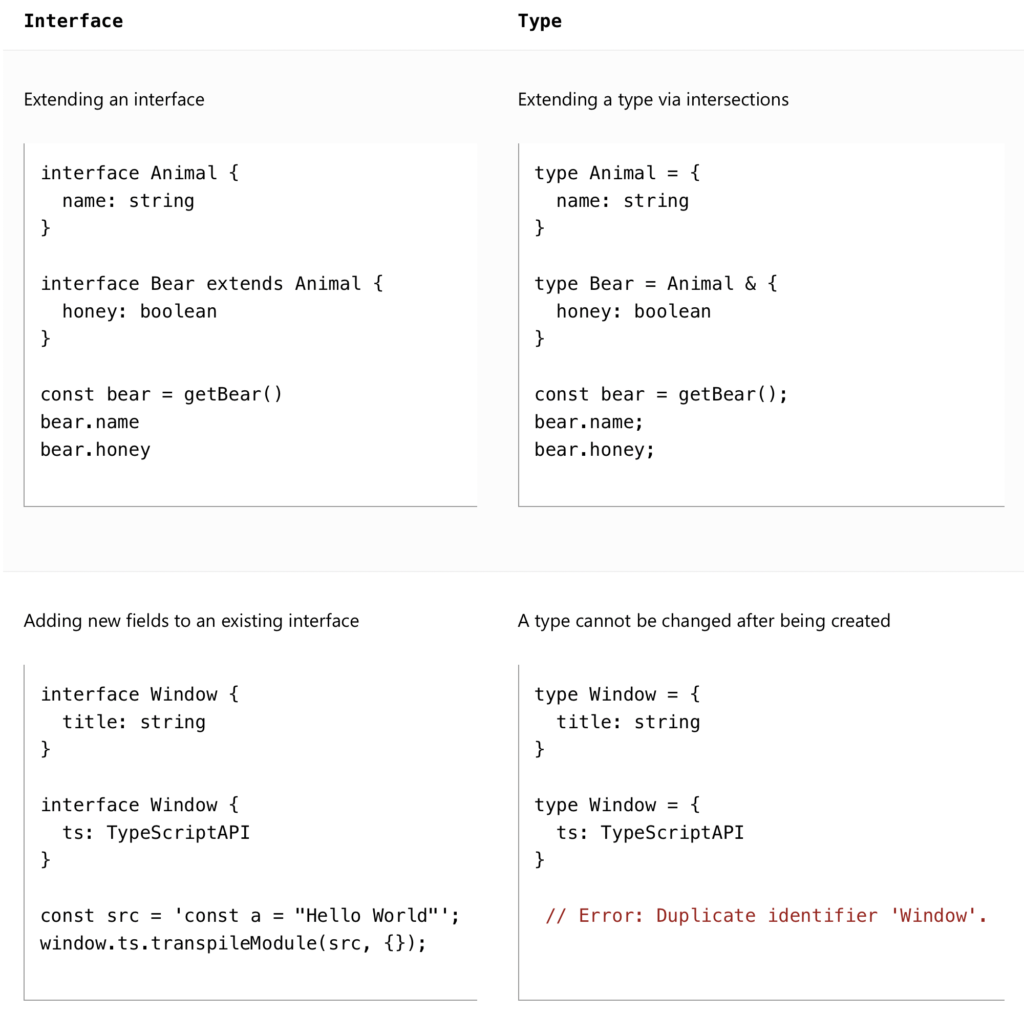
Type Assertions
const myCanvas = document.getElementById("main_canvas") as HTMLCanvasElement;
const myCanvas = <HTMLCanvasElement>document.getElementById("main_canvas");
Destructuring in declarations and assignments
Assignment
Swapping two variables can be written as a single destructuring assignment:
let x = 1;
let y = 2;
[y, x] = [x, y]; // emits y = 1 and x = 2;
Declaration
const user = {
name: 'kenan',
password: '0110010',
isAdmin: true
};
const { name, password, isAdmin } = user;
type User = {
name: string;
password: string;
isAdmin?: boolean;
};
const user: User = {
name: 'kenan',
password: '0110010'
};
const { name, password, isAdmin = false } = user;
const numbers: Array<number> = [1, 2, 3];
const [x, y, z, q = 4] = numbers;
const a1: [string, number, Date?] = ['hello', 11];
const [name, age, birthDate = new Date()] = a1;
function drawText({ text = "", location: [x, y] = [0, 0], bold = false }) {
// Draw text
}
// Call drawText with an object literal
const item = { text: "someText", location: [1, 2] as [number, number], style: "italics" };
drawText(item);