You can find more details in https://maven.apache.org/guides/getting-started/maven-in-five-minutes.html
1. Creating a Java Project with Maven
mvn archetype:generate
-DgroupId={project-package-name}
-DartifactId={project-name}
-DarchetypeArtifactId={maven-template-name}
-DinteractiveMode=false
-DarchetypeVersion=1.4
for example
mvn archetype:generate
-DgroupId=com.extuni.enum_demo4
-DartifactId=enum_demo4
-DarchetypeArtifactId=maven-archetype-quickstart
-DinteractiveMode=false
-DarchetypeVersion=1.4
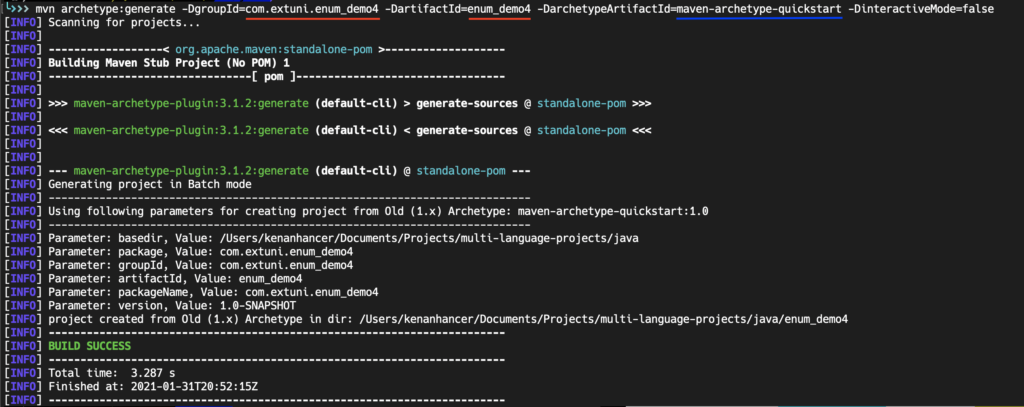
Maven Directory Layout
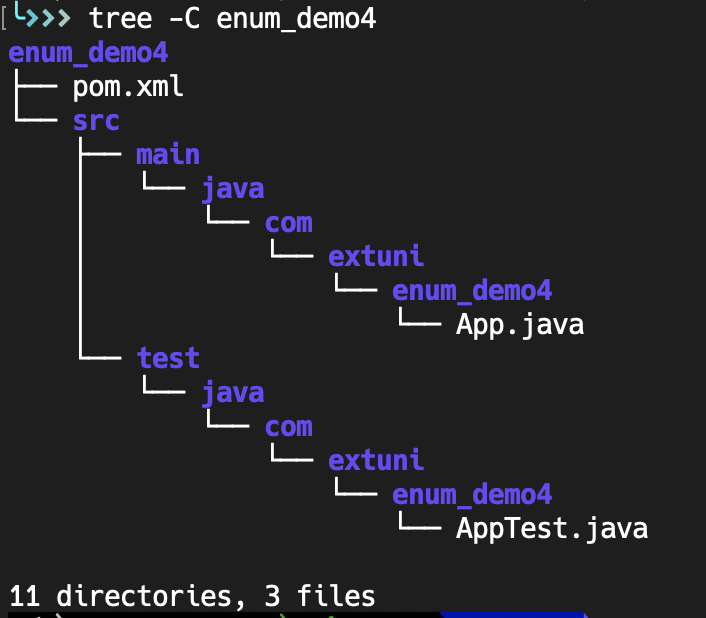
Generated App.java file content
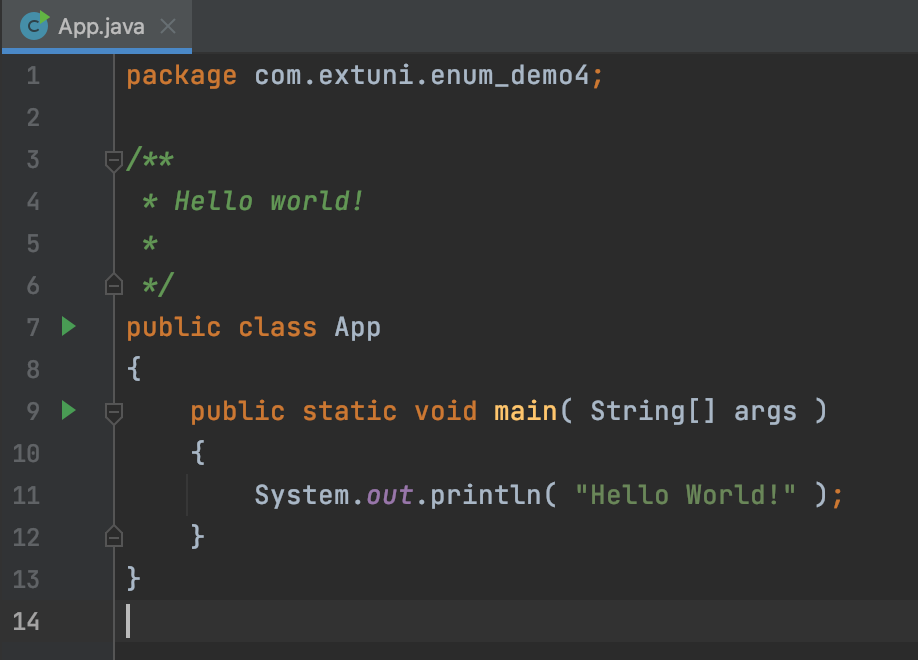
POM file
Generated pom.xml file looks like as below.
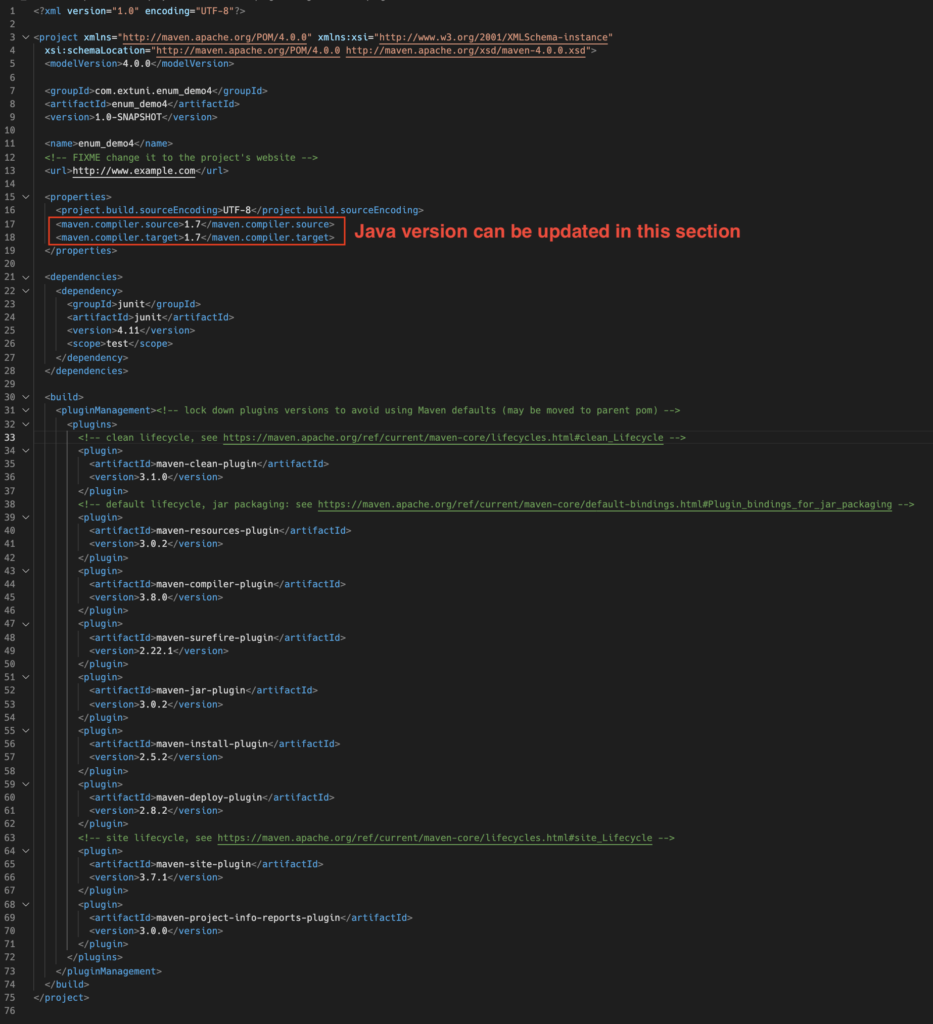
You can see updated version of pom.xml file in the following screenshot.
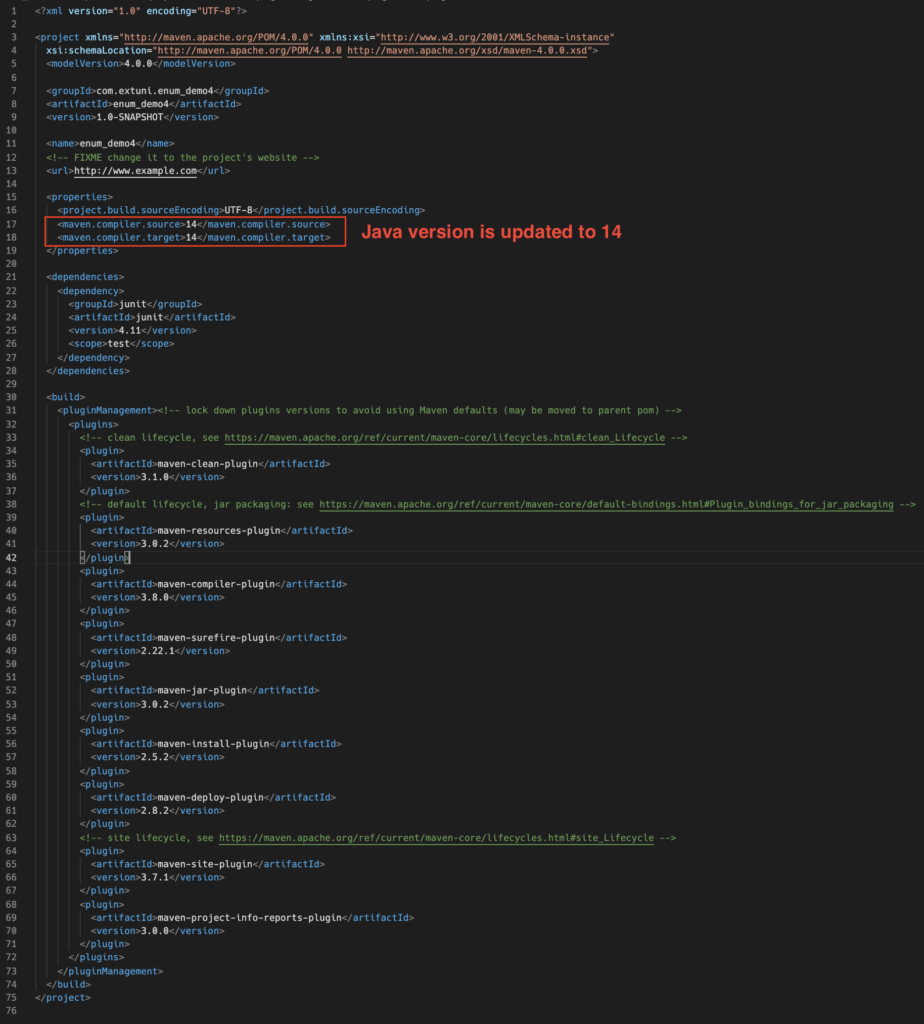
2. Compiling Java Project with Maven
mvn compile
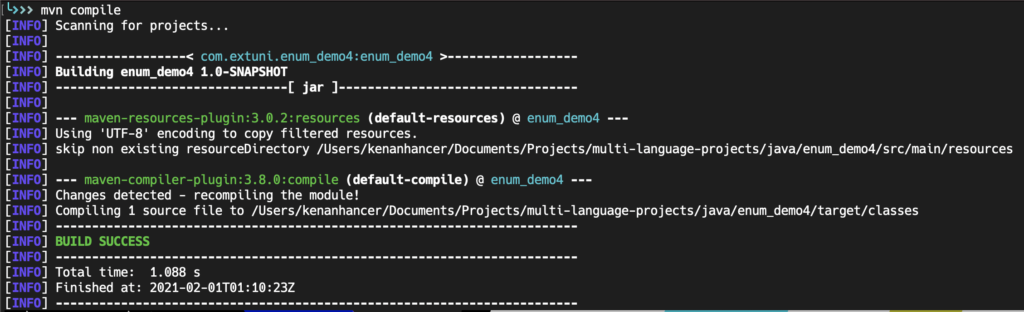
Directory layout will be changed as below.
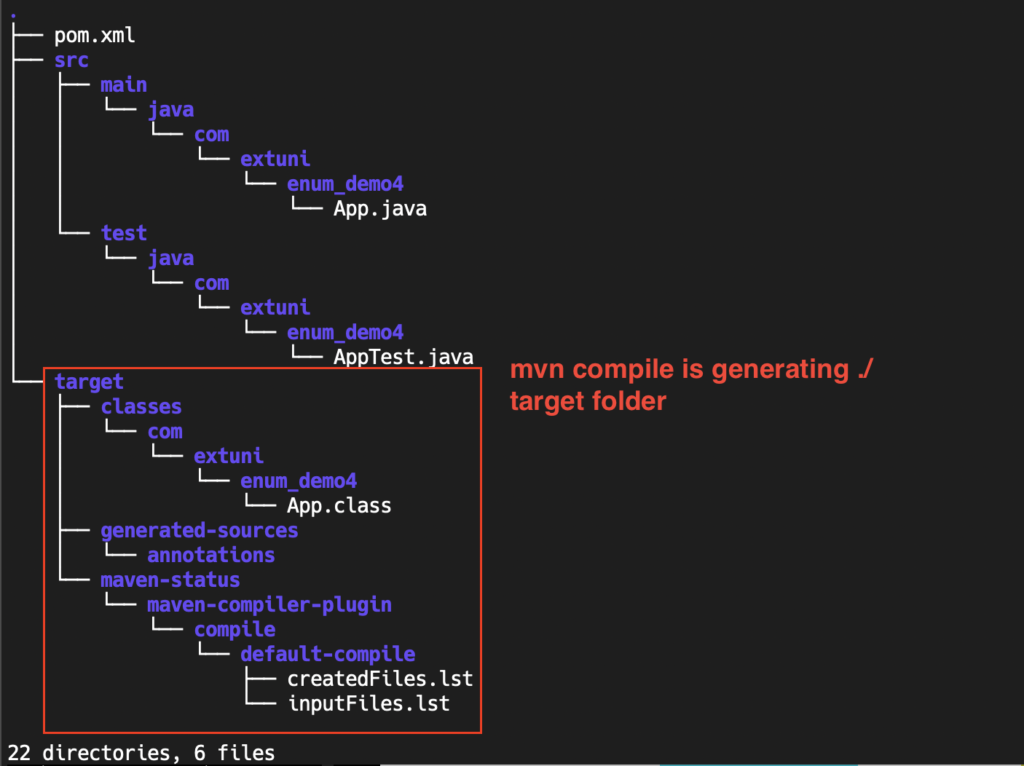
3. Cleaning Java Project with Maven
mvn clean
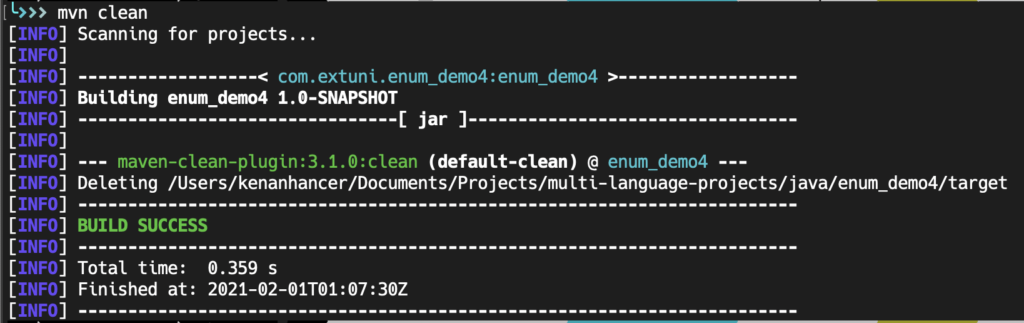
Cleaning command deletes generated target folder as shown in the following screenshot.
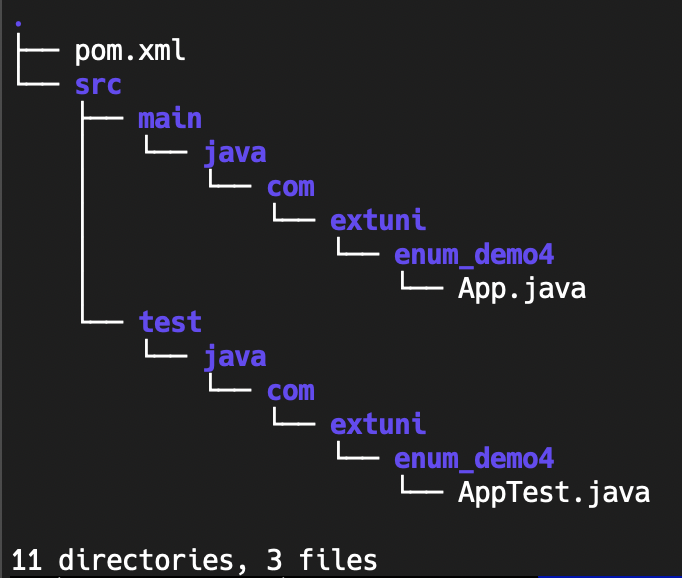
4. Compiling and running Unit tests
mvn test
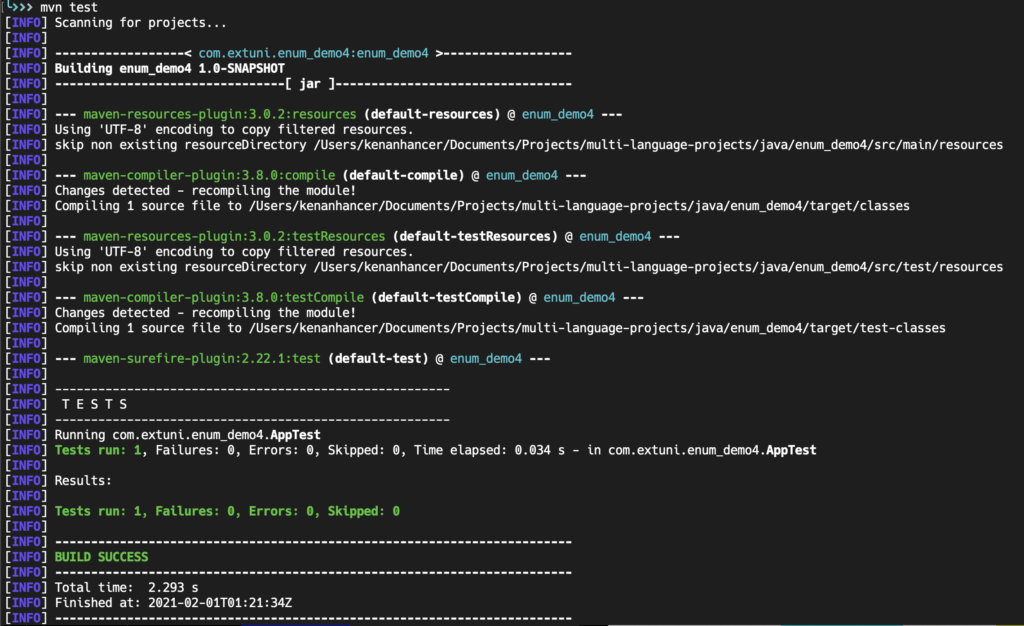
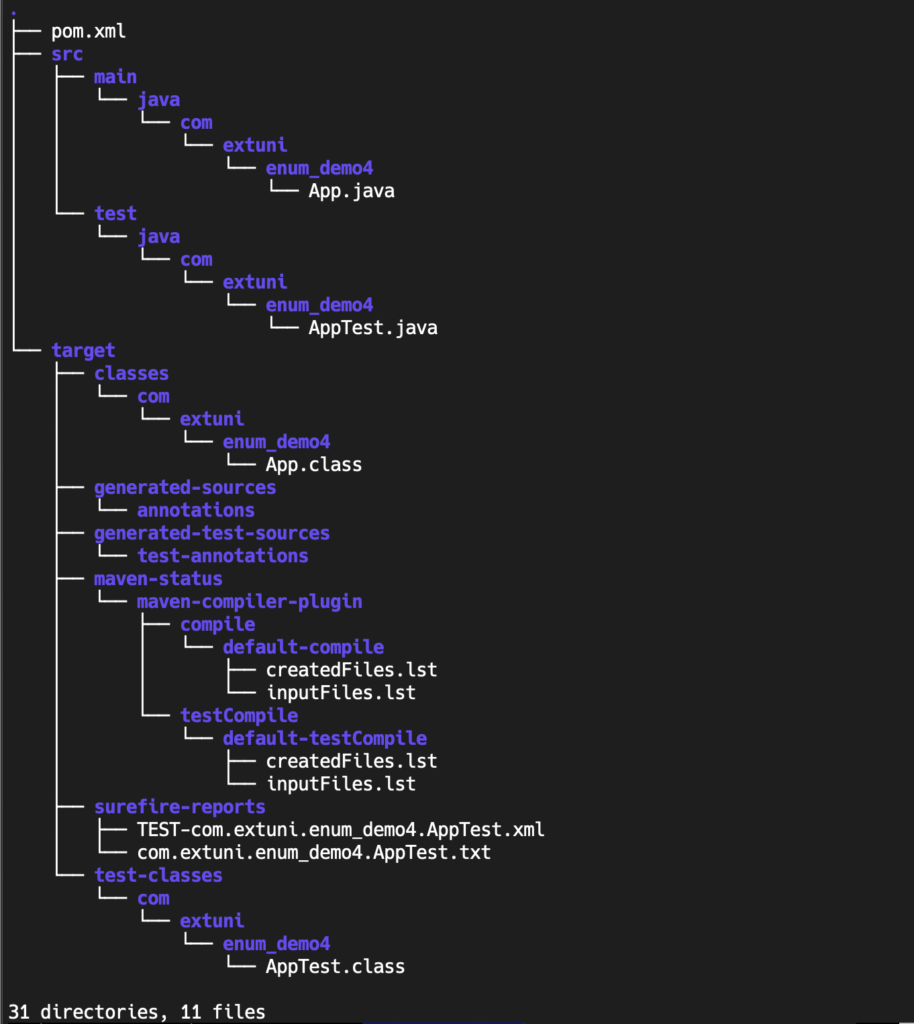
Compiling Unit Test without running
If you simply want to compile your test sources (but not execute the tests), you can execute the following:
mvn test-compile
5. Packaging Java Project with Maven
It compiles, run unit test and package the project into a jar
file and puts it into the project/target
folder.
mvn package
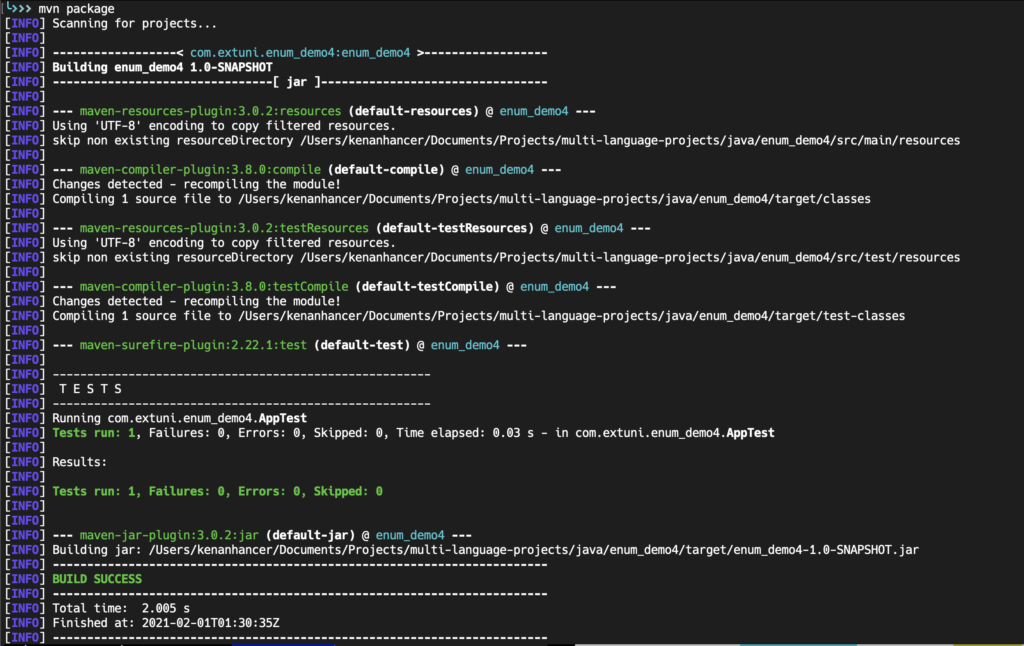
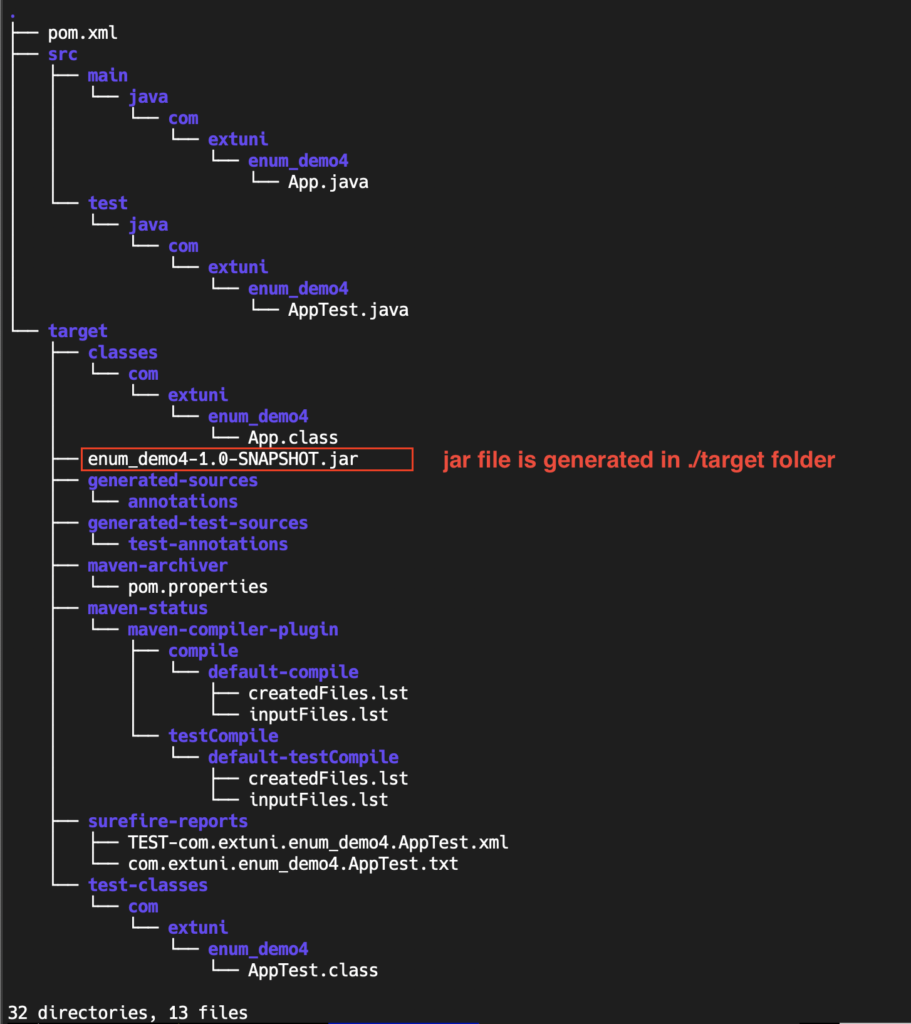
Skipping Unit Test
mvn -Dmaven.test.skip=true package
6. Installing Package in Local Maven Repository
Now you'll want to install the artifact you've generated (the JAR file) in your local repository (${user.home}/.m2/repository
is the default location).
mvn install
7. Run Java main Method with Maven
Without arguments
mvn clean compile exec:java -Dexec.mainClass="com.extuni.enum_demo4.App"
With arguments
mvn clean compile exec:java -Dexec.mainClass="com.extuni.enum_demo4.App" -Dexec.args="arg0 arg1 arg2"
Updating pom.xml file
If you don't want to specify main class full path in terminal, just update pom.xml file as the following
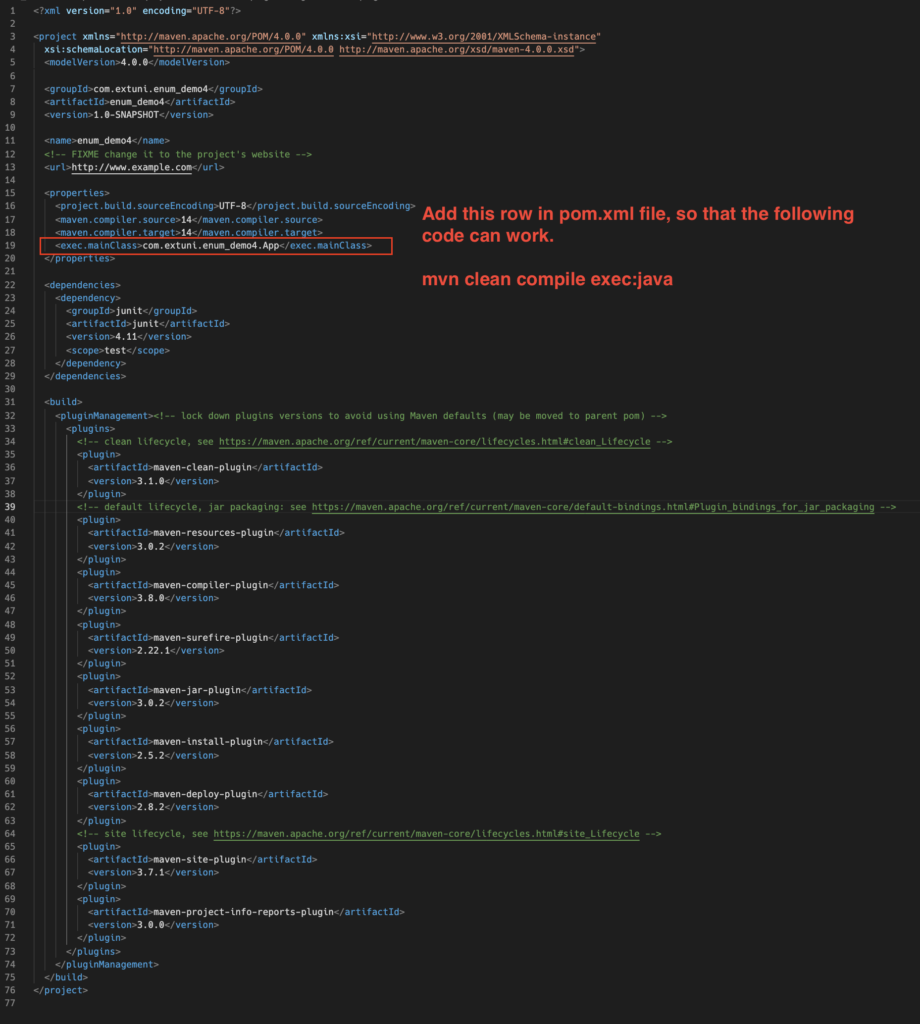
<properties>
<exec.mainClass>com.extuni.enum_demo4.App</exec.mainClass>
</properties>
mvn clean compile exec:java
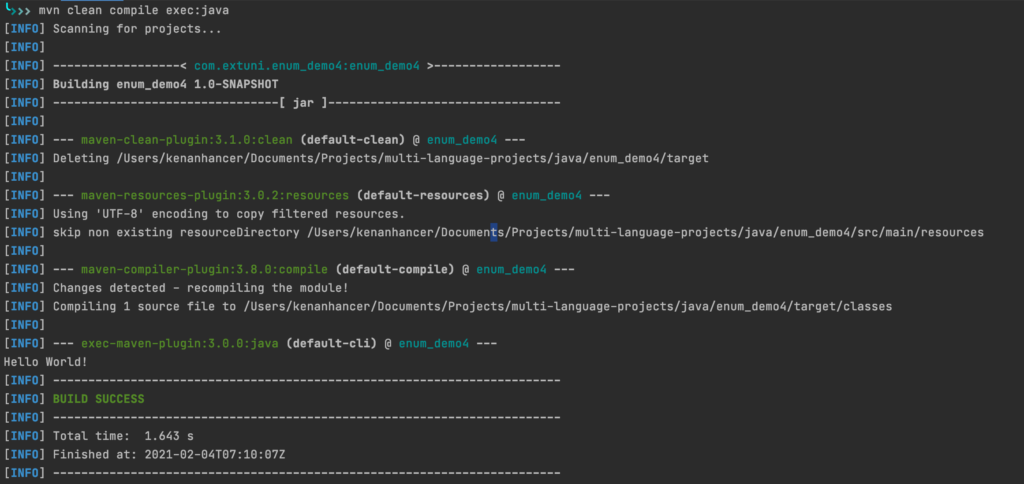
As you can see, project is cleaned, compiled and run main method. So output is Hello World! in terminal window.