I hope that somebody who forgets this kinds of basic but very important information will find this post very useful. I have demonstrated different cases of inheritance(extension) in JavaScript so that JavaScript developers can achieve OOP.
This sample code is written in different concepts you should read the following posts as well.
I try to demonstrate inheritance with ES5 in this demo. Notice that I have used function declaration and function expression in the following code examples.
After a function is created, it looks like as following picture in the run-time.
These three pictures below show that every function created like Vehicle
has common fields. Notice that screenshots show only function fields.
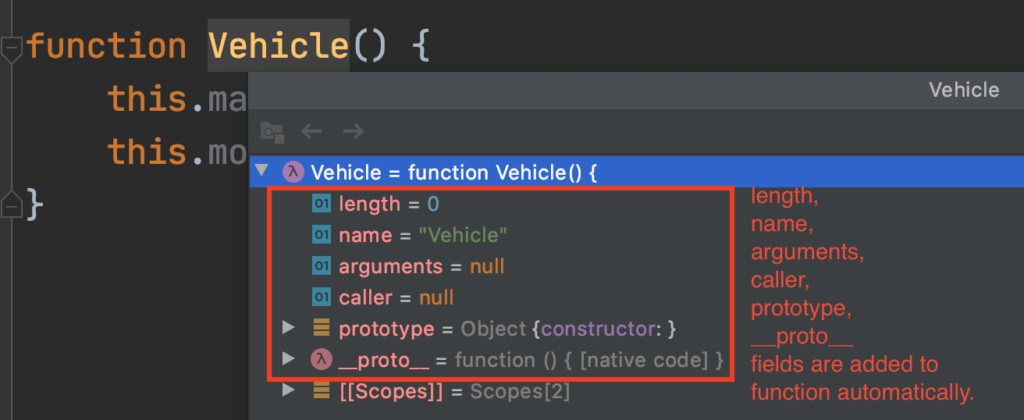
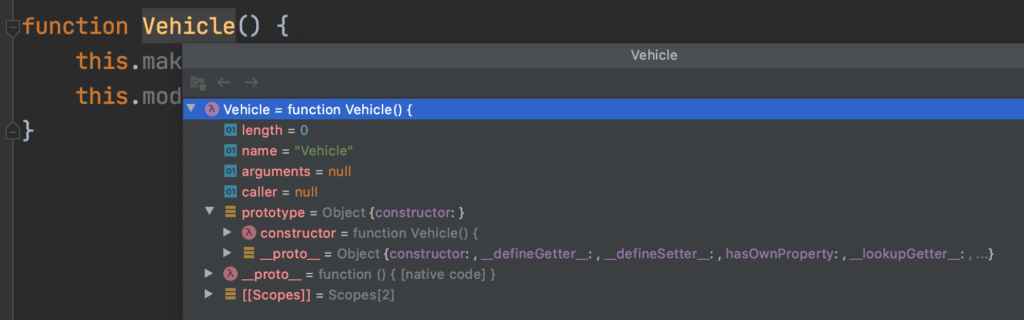
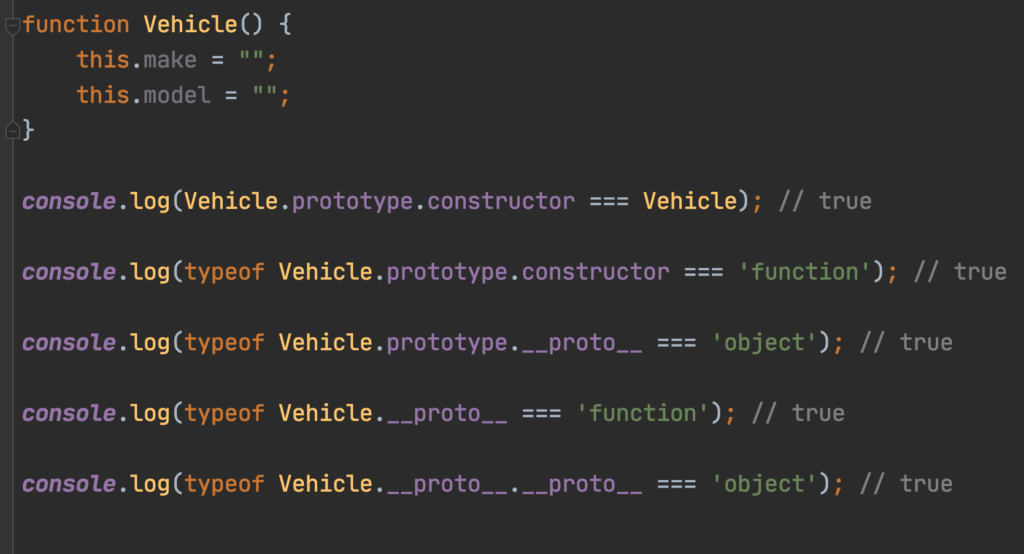
Inheritance with function expression
functions like function Person()
, function Employee()
and function Manager()
don't have any parameters but we can read value of parameters from arguments
. arguments
is a built-in function parameter.
In order to pass arguments other functions I have used function apply
method.
I updated above example code as shown below. The following code demonstrate parameterised functions with function call
method.
The only difference between function call
and apply
is that call
only accepts parameters one by one but apply
accepts parameters as an array.
Person.call(this, personId, firstName, lastName, age, gender);
Person.apply(this, arguments);