

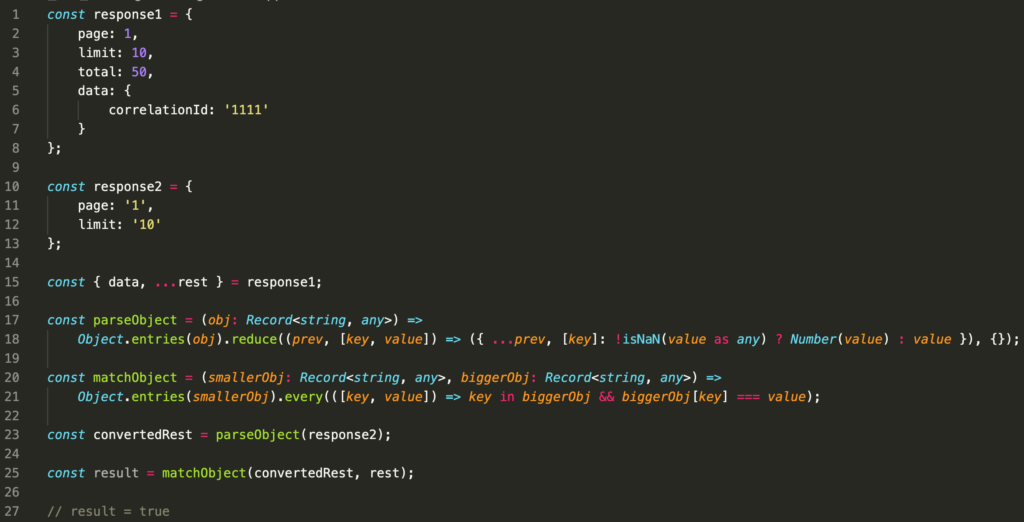
https://github.com/nodejs-projects-kenanhancer/typescript-object-oriented-programming
See the Pen TypeScriptOOPDemo1 by kenanhancer (@kenanhancer) on CodePen.
$ mkdir typescript-demo1
$ npm init -y
$ npm install --save-dev typescript @types/node rimraf
$ npx tsc --init --rootDir src --outDir build \
--esModuleInterop --resolveJsonModule --lib es6 \
--module commonjs --allowJs true --noImplicitAny true
$ mkdir src
$ echo "console.log('Hello world');" > src/index.ts
$ npx tsc
$ node dist/index.js
Continue reading See the Pen TypeScriptInterfaceDemo1 by kenanhancer (@kenanhancer) on CodePen.
Continue readingYou can see examples in the pictures and more demo codes in the rest of post.
I just want to create some demo for enum to create a builder . I improved this concept step by step in demos. Notice that all the codes are doing same job but in a different way.
You can find different usages of Type Aliases in the following demo code.
Type Aliases is defined with type
word.
Union Types is defined with the |
character to separate the different possible types.
type SelectedEvent = "Click"; // string literal type
const buttonEvent: SelectedEvent = "Click";
type Color = "Red" | "Green" | "Blue"; // string literal type with union type
const buttonForeColor: Color = "Red";
type num = 1 | 3 | 5 | 7 | 9; // number literal type with union type
type bool = true | false; // boolean literal type with union type
type TRUE = true;
type FALSE = false;
type Dictionary = {
[index: string]: any;
};
const person: Dictionary = {
"firstName": "Kenan",
"lastName": "Hancer",
"age": 36
};
interface StringArray {
[index: number]: string;
}
const names: StringArray = ["Bob", "Fred"];
let name1: string = names[0];
type obj = {success: true} | {success: false}; // object
type Result<T> = { success: true, value: T } | { success: false, error: string | number }; // object
type PersonCommonFields = { firstName: string, lastName: string };
type Person = PersonCommonFields & { isDeleted: true | false };
type Name = string; // simple type
type NameResolver = () => string; // function
type NameOrResolver = Name | NameResolver;